编写程序,创建一个实现+、-、、/和*(幂)运算的模块MyMath.py,并编写测试代码。
时间: 2024-11-06 22:13:11 浏览: 16
为了编写一个名为`MyMath.py`的模块,用于实现基本数学运算,包括加法(+), 减法(-), 乘法(*), 除法(/)以及求幂(**),你可以按照下面的方式组织:
```python
# MyMath.py
class MathOperations:
def add(self, a, b):
return a + b
def subtract(self, a, b):
return a - b
def multiply(self, a, b):
return a * b
def divide(self, a, b):
if b != 0:
return a / b
else:
raise ValueError("Cannot divide by zero.")
def power(self, a, b):
return a ** b
# 测试代码 (test_mymath.py)
import unittest
from MyMath import MathOperations
class TestMyMath(unittest.TestCase):
def setUp(self):
self.math_obj = MathOperations()
def test_addition(self):
result = self.math_obj.add(5, 3)
self.assertEqual(result, 8)
def test_subtraction(self):
result = self.math_obj.subtract(7, 4)
self.assertEqual(result, 3)
def test_multiplication(self):
result = self.math_obj.multiply(6, 2)
self.assertEqual(result, 12)
def test_division(self):
result = self.math_obj.divide(10, 2)
self.assertAlmostEqual(result, 5)
def test_power(self):
result = self.math_obj.power(2, 3)
self.assertEqual(result, 8)
#
阅读全文
相关推荐
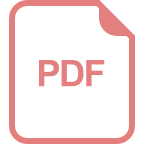

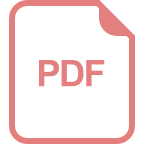
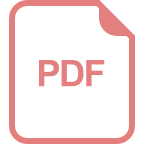
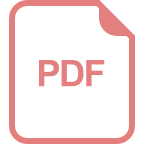
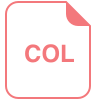
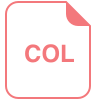
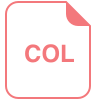
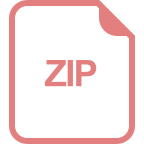
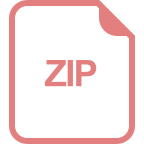
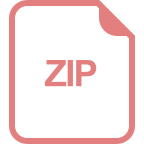
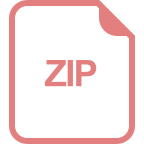
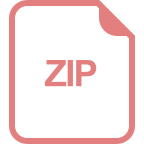
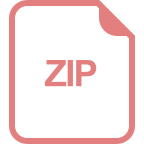