c语言:建立一个包含100个元素的数组,用随机整数填充各元素;编写两个函数实现以下功能:(1)删除指定位置的一个元素,操作点后的元素前移1个位序,第100个元素用新的随机数填充;(2)向指定位置插入一个元素,操作点(包括)后的元素后移1个位序,抛弃原第100个元素。
时间: 2024-09-18 15:02:13 浏览: 33
在C语言中,你可以通过以下几个步骤创建并操作一个包含100个元素的动态数组,并实现删除和插入的功能:
首先,你需要导入必要的头文件,并声明数组类型和一些变量,如`#include <stdio.h>`,`#include <stdlib.h>`,以及用于生成随机数的`#include <time.h>`。
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define ARRAY_SIZE 100
// 定义数组类型
typedef int array_type[ARRAY_SIZE];
// 函数原型声明
void delete_element(array_type* arr, int index);
void insert_element(array_type* arr, int index, int new_value);
int main() {
srand(time(0)); // 设置随机数种子
array_type arr = {0}; // 初始化数组为全零
// 遍历数组,用随机数填充
for (int i = 0; i < ARRAY_SIZE; i++) {
arr[i] = rand() % 100; // 生成0到99之间的随机整数
printf("%d ", arr[i]); // 打印当前元素
}
printf("\n");
// 删除指定位置的元素
int delete_index;
printf("Enter index to delete an element: ");
scanf("%d", &delete_index);
delete_element(&arr, delete_index);
// 插入新元素
int insert_value;
printf("Enter value to insert: ");
scanf("%d", &insert_value);
int insert_position;
printf("Enter position to insert: ");
scanf("%d", &insert_position);
insert_element(&arr, insert_position, insert_value);
return 0;
}
// 实现删除元素函数
void delete_element(array_type* arr, int index) {
if (index >= 0 && index < ARRAY_SIZE - 1) {
for (int i = index; i < ARRAY_SIZE - 1; i++) {
arr[i] = arr[i + 1]; // 后移元素
}
arr[ARRAY_SIZE - 1] = rand() % 100; // 用新随机数填充最后一个元素
} else {
printf("Invalid index.\n");
}
}
// 实现插入元素函数
void insert_element(array_type* arr, int index, int new_value) {
if (index >= 0 && index <= ARRAY_SIZE) {
for (int i = ARRAY_SIZE - 1; i > index; i--) {
arr[i] = arr[i - 1]; // 元素后移一位
}
arr[index] = new_value; // 插入新值
} else {
printf("Invalid position.\n");
}
}
```
运行这个程序后,你可以输入删除或插入的位置和值,然后会按照描述的操作更新数组。
阅读全文
相关推荐
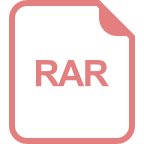
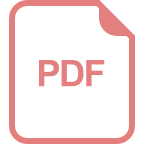
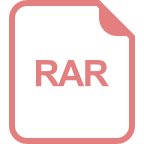

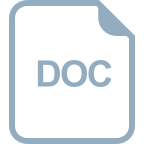
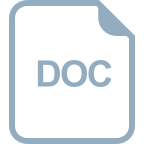
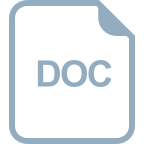
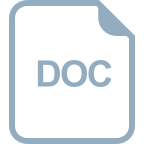










