能否提供一个C#实现工厂模式的具体代码示例?
时间: 2024-12-15 09:21:50 浏览: 11
当然可以。工厂模式是一种创建型设计模式,它用于将对象的实例化过程封装起来,隐藏其实现细节。下面是一个简单的C#工厂模式的实现示例:
```csharp
// 定义一个抽象产品接口
public interface IProduct
{
void DoSomething();
}
// 具体的产品A
public class ProductA : IProduct
{
public void DoSomething()
{
Console.WriteLine("Doing something with Product A");
}
}
// 具体的产品B
public class ProductB : IProduct
{
public void DoSomething()
{
Console.WriteLine("Doing something with Product B");
}
}
// 工厂类
public class Factory
{
// 创建产品的静态方法,可以根据需要添加更多的产品
public static IProduct CreateProduct(string type)
{
if (type == "A")
return new ProductA();
else if (type == "B")
return new ProductB();
else
throw new ArgumentException("Invalid product type.");
}
}
// 使用工厂
public class Client
{
public static void Main()
{
IProduct product = Factory.CreateProduct("A"); // 生产A产品
product.DoSomething(); // 输出: Doing something with Product A
product = Factory.CreateProduct("B"); // 生产B产品
product.DoSomething(); // 输出: Doing something with Product B
}
}
```
阅读全文
相关推荐






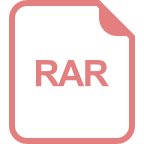
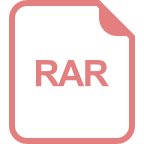


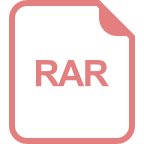
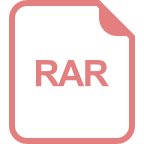
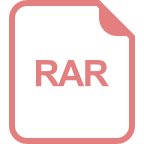



