统计字符串中的字母数,空格,数字和其他字符
时间: 2024-10-19 11:13:03 浏览: 24
统计字符串中的字母、空格、数字和其他字符通常涉及到对字符串内容进行遍历,并依据字符类别来进行计数。以下是几种常见语言的简单示例:
1. **Python**:
```python
def count_chars(s):
counts = {'letters': 0, 'spaces': 0, 'numbers': 0, 'other': 0}
for char in s:
if char.isalpha():
counts['letters'] += 1
elif char.isspace():
counts['spaces'] += 1
elif char.isdigit():
counts['numbers'] += 1
else:
counts['other'] += 1
return counts
# 示例用法
s = "Hello, World! 123"
print(count_chars(s))
```
2. **JavaScript**:
```javascript
function countChars(str) {
var counts = { letters: 0, spaces: 0, numbers: 0, other: 0 };
for (var i = 0; i < str.length; i++) {
var char = str[i];
if (/^[a-zA-Z]+$/.test(char)) {
counts.letters++;
} else if (char === ' ') {
counts.spaces++;
} else if (!isNaN(char)) {
counts.numbers++;
} else {
counts.other++;
}
}
return counts;
}
// 示例用法
let s = "Hello, World! 123";
console.log(countChars(s));
```
3. **Java**:
```java
public Map<String, Integer> countChars(String s) {
Map<String, Integer> counts = new HashMap<>();
counts.put("letters", 0);
counts.put("spaces", 0);
counts.put("numbers", 0);
counts.put("other", 0);
for (char c : s.toCharArray()) {
switch (Character.getType(c)) {
case CHAR_ALPHANUMERIC:
if (Character.isLetter(c)) counts.put("letters", counts.getOrDefault("letters", 0) + 1);
break;
case SPACE:
counts.put("spaces", counts.getOrDefault("spaces", 0) + 1);
break;
default:
counts.put("numbers" if Character.isDigit(c) else "other", counts.getOrDefault(key, 0) + 1);
}
}
return counts;
}
// 示例用法
String s = "Hello, World! 123";
System.out.println(countChars(s));
```
阅读全文
相关推荐
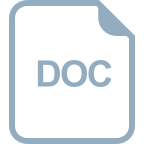
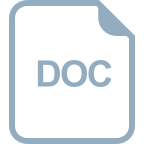
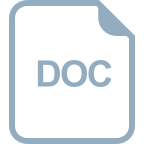















