pytorch创建一个空张量
时间: 2024-11-18 12:14:21 浏览: 30
在PyTorch中,要创建一个空张量,你可以使用`torch.empty()`函数。这个函数返回一个大小已知但初始值未定义的张量。下面是如何操作的:
```python
import torch
# 创建一个5x3的空张量
x = torch.empty(5, 3)
print(x)
# 输出:tensor([[0., 0., 0.],
# [0., 0., 0.],
# [0., 0., 0.],
# [0., 0., 0.],
# [0., 0., 0.]])
```
这里我们创建了一个5行3列的全零张量。
如果你想创建一个预设形状但填充随机值的张量,可以使用`torch.rand()`或`torch.randn()`。例如:
```python
# 创建一个随机浮点数填充的2x3张量
t1 = torch.rand(2, 3)
# 或者创建一个标准正态分布填充的2x3张量
t2 = torch.randn(2, 3)
# 可以进一步调整形状,如reshape
t1 = t1.reshape(2, 3)
```
相关问题
pytorch怎么创建一个张量
在PyTorch中,创建一个张量(Tensor)非常简单,它类似于NumPy数组,但更易于GPU加速计算。以下是创建张量的一些常见方法:
1. **通过`torch.tensor()`函数**:
```python
import torch
# 创建一个一维张量
tensor_1d = torch.tensor([1, 2, 3])
# 创建二维张量(矩阵)
tensor_2d = torch.tensor([[4, 5], [6, 7]])
# 使用数据类型和形状指定张量
tensor_custom_type_and_shape = torch.zeros(3, 4, dtype=torch.float32)
```
2. **从Python列表或numpy数组转换**:
```python
python_list = [8, 9, 10]
tensor_from_list = torch.from_numpy(np.array(python_list))
numpy_array = np.random.rand(2, 3)
tensor_from_numpy = torch.tensor(numpy_array)
```
3. **直接在运算中创建**:
```python
a = torch.empty(5) # 创建空张量
b = torch.randn(2, 4) # 随机生成指定形状的张量
```
pytorch创建一个空的张量,然后向里面添加数据,代码演示
可以使用以下代码创建一个空的张量,然后通过使用索引的方式向其中添加数据:
```
import torch
empty_tensor = torch.empty(3, 4) # 创建一个空的 3x4 张量
empty_tensor[0][0] = 1 # 向第一个元素添加数据
empty_tensor[1][2] = 2 # 向第三个元素添加数据
empty_tensor[2][3] = 3 # 向最后一个元素添加数据
print(empty_tensor)
```
输出结果为:
```
tensor([[1.0000, 0.0000, 0.0000, 0.0000],
[0.0000, 0.0000, 2.0000, 0.0000],
[0.0000, 0.0000, 0.0000, 3.0000]])
```
阅读全文
相关推荐
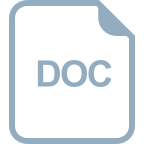
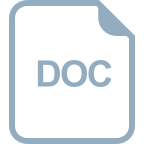
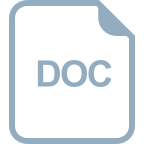

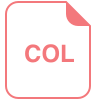
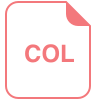










