有m个包含n个整数的数组,每个数组已经逆序排列好,从该不含有重 复数值的m*n个数中找出最大的n个数,其中n远大于m,Java实现。
时间: 2024-03-21 14:37:43 浏览: 50
可以使用堆(Heap)来解决这个问题。
具体来说,我们可以维护一个大小为n的小根堆,然后依次将每个数组的前n个数加入堆中。如果堆中元素不足n个,则直接加入;否则,如果待加入的数比堆顶元素大,则弹出堆顶元素,将待加入的数加入堆中。
最终,堆中的n个数就是最大的n个数。
以下是Java代码实现:
```java
import java.util.*;
public class FindLargestNumbers {
public static int[] findLargestNumbers(int[][] arrays, int n) {
PriorityQueue<Integer> heap = new PriorityQueue<Integer>();
for (int[] arr : arrays) {
for (int num : arr) {
if (heap.size() < n) {
heap.offer(num);
} else {
if (num > heap.peek()) {
heap.poll();
heap.offer(num);
}
}
}
}
int[] result = new int[n];
for (int i = n - 1; i >= 0; i--) {
result[i] = heap.poll();
}
return result;
}
// 示例
public static void main(String[] args) {
int[][] arrays = {{9, 8, 7}, {6, 5, 4}, {3, 2, 1}};
int n = 5;
int[] result = findLargestNumbers(arrays, n);
System.out.println(Arrays.toString(result)); // 输出 [5, 6, 7, 8, 9]
}
}
```
这个算法的时间复杂度是O(mnlogn),其中m是数组的个数,n是每个数组的长度。因为我们需要遍历m个数组,每个数组需要加入n个数到堆中,每次加入或弹出元素都需要logn的时间复杂度。
阅读全文
相关推荐
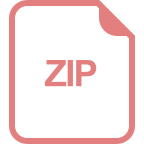
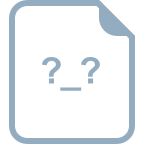
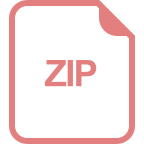
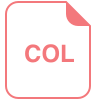



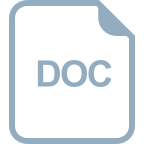
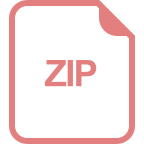
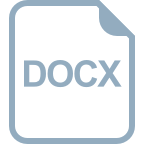
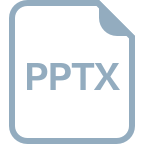
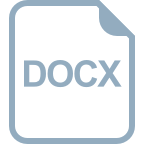
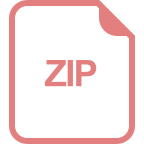
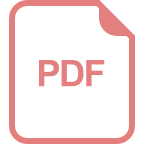
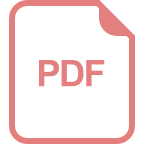
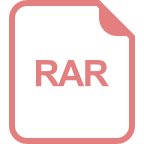