c语言实现usb接口协议源码
时间: 2023-08-03 08:07:38 浏览: 64
实现 USB 接口协议需要用到 USB 主机控制器和 USB 设备控制器的驱动程序,这些驱动程序需要直接与硬件进行交互。因此,编写 USB 接口协议的源码需要深入了解 USB 协议和硬件驱动程序的开发。
以下是一个简单的 C 语言实现 USB 接口协议源码示例,仅供参考:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdint.h>
#include <stdbool.h>
#include <usb.h>
#define VENDOR_ID 0x1234
#define PRODUCT_ID 0x5678
#define ENDPOINT_IN 0x81
#define ENDPOINT_OUT 0x01
#define MAX_PACKET_SIZE 64
struct usb_dev_handle *open_device(void){
struct usb_bus *bus;
struct usb_device *dev;
struct usb_dev_handle *handle = NULL;
usb_init();
usb_find_busses();
usb_find_devices();
for(bus = usb_get_busses(); bus; bus = bus->next){
for(dev = bus->devices; dev; dev = dev->next){
if(dev->descriptor.idVendor == VENDOR_ID && dev->descriptor.idProduct == PRODUCT_ID){
handle = usb_open(dev);
break;
}
}
if(handle) break;
}
if(!handle){
fprintf(stderr, "Device not found\n");
exit(1);
}
if(usb_set_configuration(handle, 1) < 0){
fprintf(stderr, "Could not set configuration\n");
usb_close(handle);
exit(1);
}
if(usb_claim_interface(handle, 0) < 0){
fprintf(stderr, "Could not claim interface\n");
usb_close(handle);
exit(1);
}
return handle;
}
void close_device(struct usb_dev_handle *handle){
usb_release_interface(handle, 0);
usb_close(handle);
}
void send_data(struct usb_dev_handle *handle, uint8_t *data, uint32_t size){
int transferred = usb_bulk_write(handle, ENDPOINT_OUT, data, size, 1000);
if(transferred < 0){
fprintf(stderr, "Error sending data: %s\n", usb_strerror());
exit(1);
}
}
void receive_data(struct usb_dev_handle *handle, uint8_t *data, uint32_t size){
int transferred = usb_bulk_read(handle, ENDPOINT_IN, data, size, 1000);
if(transferred < 0){
fprintf(stderr, "Error receiving data: %s\n", usb_strerror());
exit(1);
}
}
int main(int argc, char **argv){
struct usb_dev_handle *handle;
uint8_t buf[MAX_PACKET_SIZE];
uint32_t size;
handle = open_device();
// Send some data
memset(buf, 0x55, MAX_PACKET_SIZE);
send_data(handle, buf, MAX_PACKET_SIZE);
// Receive some data
receive_data(handle, buf, MAX_PACKET_SIZE);
size = usb_get_max_packet_size(handle, ENDPOINT_IN);
printf("Received %d bytes of data\n", size);
close_device(handle);
return 0;
}
```
该示例使用 libusb 库进行 USB 设备操作,实现了 USB 数据的发送和接收。其中,`open_device` 函数用于打开 USB 设备,`close_device` 函数用于关闭设备,`send_data` 函数用于发送数据,`receive_data` 函数用于接收数据。具体实现过程中,需要根据硬件和协议的要求进行相应的配置和处理。
相关推荐
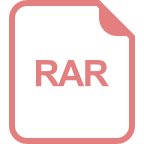
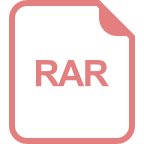
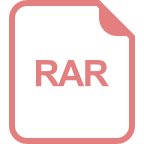
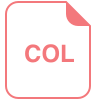
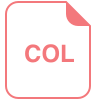
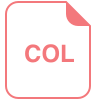
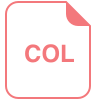
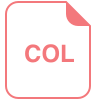






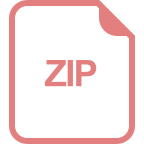
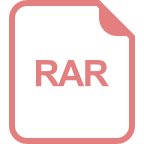
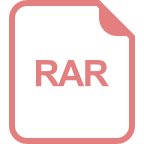
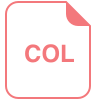