android中RoundImageView如何实现鼠标进入时和退出时显示不同效果
时间: 2024-05-06 18:19:37 浏览: 13
Android 中的 RoundImageView 是一个自定义 View,可以根据需求自己实现鼠标进入时和退出时显示不同效果的功能。
以下是一个实现鼠标进入时和退出时显示不同效果的 RoundImageView 的示例代码:
```
public class RoundImageView extends ImageView {
private int mBorderColor = Color.RED;
private int mBorderWidth = 0;
private int mRadius = 0;
public RoundImageView(Context context) {
super(context);
}
public RoundImageView(Context context, AttributeSet attrs) {
super(context, attrs);
initAttrs(context, attrs);
}
public RoundImageView(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
initAttrs(context, attrs);
}
private void initAttrs(Context context, AttributeSet attrs) {
TypedArray a = context.obtainStyledAttributes(attrs, R.styleable.RoundImageView);
mBorderColor = a.getColor(R.styleable.RoundImageView_borderColor, Color.RED);
mBorderWidth = a.getDimensionPixelSize(R.styleable.RoundImageView_borderWidth, 0);
mRadius = a.getDimensionPixelSize(R.styleable.RoundImageView_radius, 0);
a.recycle();
}
@Override
protected void onDraw(Canvas canvas) {
Drawable drawable = getDrawable();
if (drawable == null) {
return;
}
if (getWidth() == 0 || getHeight() == 0) {
return;
}
Bitmap b = ((BitmapDrawable) drawable).getBitmap();
if (b == null) {
return;
}
Bitmap bitmap = b.copy(Bitmap.Config.ARGB_8888, true);
int w = getWidth(), h = getHeight();
Bitmap roundBitmap = getCroppedBitmap(bitmap, w, h);
canvas.drawBitmap(roundBitmap, 0, 0, null);
if (mBorderWidth > 0) {
Paint paint = new Paint();
paint.setColor(mBorderColor);
paint.setStyle(Paint.Style.STROKE);
paint.setStrokeWidth(mBorderWidth);
canvas.drawCircle(w / 2, h / 2, mRadius - mBorderWidth / 2, paint);
}
}
private Bitmap getCroppedBitmap(Bitmap bmp, int radius, int border) {
Bitmap sbmp;
if (bmp.getWidth() != radius || bmp.getHeight() != radius) {
float smallest = Math.min(bmp.getWidth(), bmp.getHeight());
float factor = smallest / radius;
sbmp = Bitmap.createScaledBitmap(bmp, (int) (bmp.getWidth() / factor), (int) (bmp.getHeight() / factor), false);
} else {
sbmp = bmp;
}
Bitmap output = Bitmap.createBitmap(radius, radius, Bitmap.Config.ARGB_8888);
Canvas canvas = new Canvas(output);
int color = Color.WHITE;
Paint paint = new Paint();
Rect rect = new Rect(0, 0, radius, radius);
paint.setAntiAlias(true);
paint.setColor(color);
canvas.drawARGB(0, 0, 0, 0);
canvas.drawCircle(radius / 2, radius / 2, mRadius, paint);
paint.setXfermode(new PorterDuffXfermode(PorterDuff.Mode.SRC_IN));
canvas.drawBitmap(sbmp, rect, rect, paint);
return output;
}
@Override
public boolean onTouchEvent(MotionEvent event) {
switch (event.getAction()) {
case MotionEvent.ACTION_DOWN:
// 鼠标进入时的处理
mBorderColor = Color.BLUE;
mBorderWidth = 10;
invalidate();
break;
case MotionEvent.ACTION_UP:
// 鼠标退出时的处理
mBorderColor = Color.RED;
mBorderWidth = 0;
invalidate();
break;
default:
break;
}
return super.onTouchEvent(event);
}
}
```
在 RoundImageView 中,我们重写了 onTouchEvent 方法,当鼠标进入时修改了边框的颜色和宽度,在鼠标退出时则恢复为原来的颜色和宽度。这样就实现了鼠标进入时和退出时显示不同效果的功能。
相关推荐
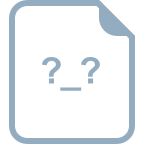
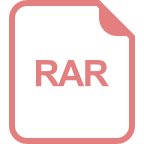
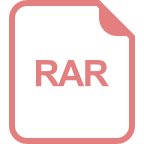














