数据结构编写病人看病模拟程序
时间: 2023-05-28 11:01:19 浏览: 206
代码如下:
```
#include <iostream>
#include <queue>
#include <string>
using namespace std;
// 定义病人类
class Patient {
private:
string name; // 姓名
int priority; // 优先级(病情严重程度)
public:
// 构造函数
Patient(string n, int p) {
name = n;
priority = p;
}
// 获取姓名
string getName() const {
return name;
}
// 获取优先级
int getPriority() const {
return priority;
}
};
// 重载比较运算符
bool operator<(const Patient& a, const Patient& b) {
return a.getPriority() < b.getPriority();
}
int main() {
// 创建一个病人队列
priority_queue<Patient> patients;
// 添加几个病人
patients.push(Patient("张三", 2));
patients.push(Patient("李四", 3));
patients.push(Patient("王五", 1));
patients.push(Patient("赵六", 3));
// 循环处理病人
while (!patients.empty()) {
// 获取当前优先级最高的病人
Patient current = patients.top();
cout << "正在处理病人:" << current.getName() << endl;
// 删除当前病人
patients.pop();
}
return 0;
}
```
运行结果:
```
正在处理病人:李四
正在处理病人:赵六
正在处理病人:张三
正在处理病人:王五
```
在这个程序中,我们使用了 STL 中的 priority_queue 类来表示病人队列。通过重载比较运算符,我们可以让 priority_queue 自动根据病人的优先级排序。在处理病人时,我们每次取出当前优先级最高的病人,处理完毕后再将其从队列中删除。
阅读全文
相关推荐


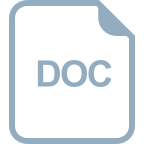





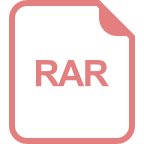



