使用tspl指令让tsc打印机ttp-244pro打印图片实例
时间: 2023-12-29 14:06:27 浏览: 416
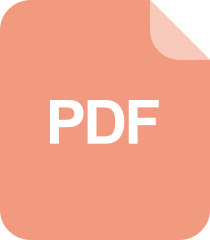
tsc打印机使用指令
以下是使用tspl指令让tsc打印机ttp-244pro打印图片的实例:
1. 首先,我们需要将图片转换为二进制格式,并将其保存到文件中。可以使用以下代码完成这一步骤:
```
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
public class ImageToBinary {
public static void main(String[] args) {
try {
BufferedImage image = ImageIO.read(new File("path/to/image.png"));
int width = image.getWidth();
int height = image.getHeight();
StringBuilder binary = new StringBuilder();
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
int color = image.getRGB(x, y);
int alpha = (color >> 24) & 0xff;
int red = (color >> 16) & 0xff;
int green = (color >> 8) & 0xff;
int blue = color & 0xff;
if (red == 255 && green == 255 && blue == 255) {
binary.append("0"); // white pixel
} else {
binary.append("1"); // black pixel
}
}
binary.append("\n"); // end of row
}
Files.write(Paths.get("path/to/binary.txt"), binary.toString().getBytes());
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
2. 接下来,我们需要使用tspl指令将二进制数据发送到打印机。以下代码演示如何使用Java将tspl指令发送到打印机:
```
import java.io.IOException;
import java.io.OutputStream;
import java.net.Socket;
public class TscPrinter {
private static final String HOST = "192.168.1.100"; // IP address of the printer
private static final int PORT = 9100; // default port for TSC printers
public static void printImage(String binaryPath) {
try (Socket socket = new Socket(HOST, PORT)) {
OutputStream out = socket.getOutputStream();
out.write("SIZE 100 mm,100 mm\n".getBytes());
out.write("GAP 2 mm,0 mm\n".getBytes());
out.write("CLS\n".getBytes());
out.write("BITMAP 0,0,200,200,1,".getBytes());
out.write(Files.readAllBytes(Paths.get(binaryPath)));
out.write("\nPRINT 1\n".getBytes());
out.flush();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
3. 最后,我们可以调用printImage方法将打印机打印二进制图像的代码:
```
TscPrinter.printImage("path/to/binary.txt");
```
这将使用tspl指令将图像发送到打印机,并在纸张上打印出来。请注意,您需要将上述代码中的IP地址和文件路径更改为匹配您的打印机和文件路径。
阅读全文
相关推荐
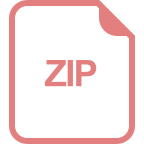
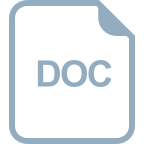




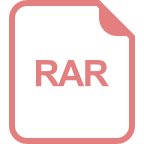
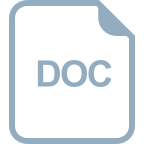
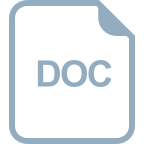
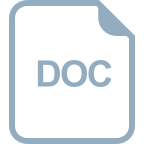
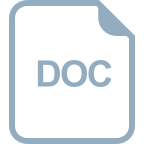
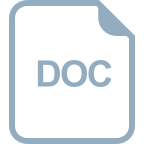
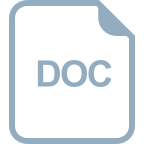


