vue中通过get请求怎么将json数据存入mysql数据库 详细代码
时间: 2023-06-16 18:06:46 浏览: 60
Vue.js 是一个前端框架,不能直接将数据存入 MySQL 数据库。如果你的需求是前端发送 GET 请求获取到的数据存入 MySQL 数据库,那么你需要一个后端服务器来处理这个请求。
以下是一个简单的示例代码,假设你的后端服务器使用 Node.js 和 Express 框架:
```
// index.js
const express = require('express')
const mysql = require('mysql')
const app = express()
// 创建 MySQL 连接池
const pool = mysql.createPool({
host: 'localhost',
user: 'root',
password: 'password',
database: 'database_name'
})
// 处理 GET 请求
app.get('/data', (req, res) => {
// 从 MySQL 连接池中获取一个连接
pool.getConnection((err, connection) => {
if (err) {
console.error(err)
res.status(500).send('Internal Server Error')
} else {
// 从 URL 中获取数据
const data = req.query.data
// 执行 MySQL 查询
connection.query('INSERT INTO table_name (column_name) VALUES (?)', [data], (err, results) => {
connection.release() // 释放连接
if (err) {
console.error(err)
res.status(500).send('Internal Server Error')
} else {
res.send('Data inserted successfully')
}
})
}
})
})
// 启动服务器
app.listen(3000, () => {
console.log('Server started on http://localhost:3000')
})
```
在上面的示例代码中,我们创建了一个 Express 应用,并通过 `mysql.createPool` 方法创建了一个 MySQL 连接池。当用户访问 `/data` 路由时,我们从 URL 中获取数据,然后执行 MySQL 插入操作,最后返回结果给用户。
在你的 Vue.js 应用中,你可以使用 `axios` 库发送 GET 请求,示例代码如下:
```
// 在 Vue.js 中发送 GET 请求
axios.get('/data', {
params: {
data: 'hello world'
}
}).then(response => {
console.log(response.data)
}).catch(error => {
console.error(error)
})
```
在上面的示例代码中,我们使用 `axios.get` 方法发送 GET 请求,并将数据作为 URL 参数传递。当服务器响应时,我们通过 `then` 方法处理响应数据,或通过 `catch` 方法处理错误。
相关推荐
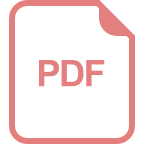
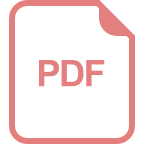
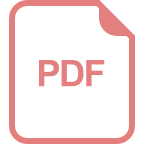














