java编写话费统计的MR程序,计算每个电话号码的总流量,包括上行流量,下行流量。文件格式为key + "\t" + data[0] + "\t" + data[1] + "\t" + data[2] + "\t" + data[3]
时间: 2024-03-25 21:41:12 浏览: 18
好的,这是一个典型的MapReduce程序,下面是一个简单的Java实现过程:
1. Map阶段
```java
public static class Map extends Mapper<LongWritable, Text, Text, Text> {
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String[] data = value.toString().split("\t"); // 拆分数据
String phone_number = data[1]; // 获取电话号码
int up_flow = Integer.parseInt(data[2]); // 获取上行流量
int down_flow = Integer.parseInt(data[3]); // 获取下行流量
context.write(new Text(phone_number), new Text(up_flow + "\t" + down_flow)); // 输出为(key, value)的形式
}
}
```
2. Reduce阶段
```java
public static class Reduce extends Reducer<Text, Text, Text, Text> {
public void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
int total_up_flow = 0;
int total_down_flow = 0;
for (Text value : values) {
String[] data = value.toString().split("\t"); // 拆分数据
total_up_flow += Integer.parseInt(data[0]); // 累加上行流量
total_down_flow += Integer.parseInt(data[1]); // 累加下行流量
}
context.write(key, new Text(total_up_flow + "\t" + total_down_flow)); // 输出为(key, value)的形式
}
}
```
最终的输出结果为每个电话号码对应的总流量数据。
如果需要考虑数据压缩和优化等问题,可以使用序列化和压缩技术,以及调整MapReduce的参数等手段来提高程序的性能和效率。
相关推荐
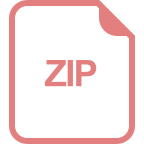
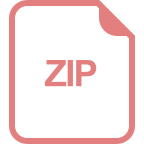
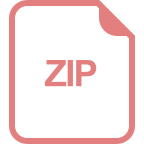














