keil中怎样初始化malloc的heap
时间: 2023-11-08 17:05:43 浏览: 99
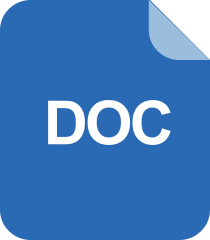
Keil MDK下如何设置非零初始化变量.doc
在 Keil 中,使用 `malloc` 函数进行内存分配时,需要为其提供一个堆空间,否则会出现内存分配失败等问题。以下是在 Keil 中初始化堆空间的步骤:
1. 首先在代码中定义堆空间的起始地址和大小,例如:
```c
#define HEAP_START 0x20000000
#define HEAP_SIZE 0x1000
```
这里定义了堆空间的起始地址为 `0x20000000`,大小为 `0x1000` 字节。
2. 在代码中添加 `malloc` 函数的实现。可以使用 Keil 提供的 `rt_misc.c` 文件中的 `__user_heap_extend` 函数作为 `malloc` 函数的实现,例如:
```c
#include <stddef.h>
#include <stdint.h>
#define HEAP_START 0x20000000
#define HEAP_SIZE 0x1000
static uint8_t heap[HEAP_SIZE] __attribute__((section(".heap")));
void* __user_heap_extend(size_t incr) {
static uint8_t* heap_end = NULL;
uint8_t* prev_heap_end;
if (heap_end == NULL) {
heap_end = heap;
}
prev_heap_end = heap_end;
if (heap_end + incr > heap + HEAP_SIZE) {
// 内存不足,返回 NULL
return NULL;
}
heap_end += incr;
return prev_heap_end;
}
void* malloc(size_t size) {
return __user_heap_extend(size);
}
void free(void* ptr) {
// 空函数,不需要实现
}
```
这里使用 `__attribute__((section(".heap")))` 将 `heap` 数组放在 `.heap` 段中,以便在链接时将其放置在指定的地址。
3. 在代码中调用 `malloc` 函数进行内存分配,例如:
```c
int main() {
int* p = (int*) malloc(sizeof(int));
if (p == NULL) {
// 内存分配失败
return 1;
}
// 使用分配的内存
*p = 123;
// 释放内存
free(p);
return 0;
}
```
这样就完成了在 Keil 中初始化堆空间的操作,可以使用 `malloc` 函数进行动态内存分配了。需要注意的是,由于 `malloc` 函数实现中使用了静态变量,因此需要在 `__user_heap_extend` 函数中将其声明为 `static` 变量,以避免链接时出现重复定义的错误。
阅读全文
相关推荐
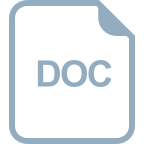
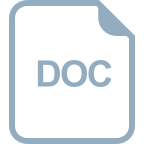
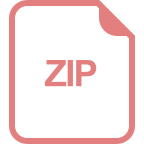
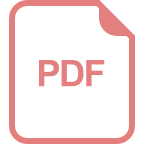
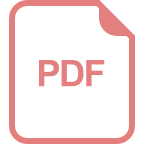
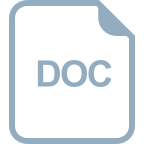
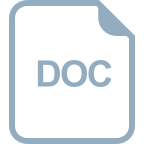
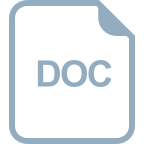
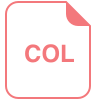
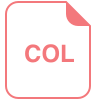
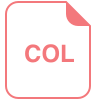
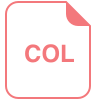
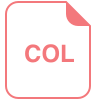




