ffmpeg如何解码H265视频流,请使用代码举例并解释
时间: 2023-06-19 16:08:13 浏览: 193
使用FFmpeg解码H.265视频流的步骤如下:
1. 打开输入流并获取输入格式上下文
```c
AVFormatContext *pFormatCtx = NULL;
if (avformat_open_input(&pFormatCtx, input_file, NULL, NULL) != 0) {
fprintf(stderr, "Failed to open input file %s\n", input_file);
return -1;
}
if (avformat_find_stream_info(pFormatCtx, NULL) < 0) {
fprintf(stderr, "Failed to retrieve input stream information\n");
return -1;
}
```
2. 找到视频流并获取视频解码器
```c
int video_stream_idx = -1;
AVCodecParameters *pCodecParams = NULL;
AVCodec *pCodec = NULL;
for (int i = 0; i < pFormatCtx->nb_streams; i++) {
if (pFormatCtx->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO) {
video_stream_idx = i;
pCodecParams = pFormatCtx->streams[i]->codecpar;
pCodec = avcodec_find_decoder(pCodecParams->codec_id);
break;
}
}
if (video_stream_idx == -1) {
fprintf(stderr, "Failed to find video stream\n");
return -1;
}
if (!pCodec) {
fprintf(stderr, "Failed to find decoder for codec ID %d\n", pCodecParams->codec_id);
return -1;
}
```
3. 打开解码器并分配解码器上下文
```c
AVCodecContext *pCodecCtx = avcodec_alloc_context3(pCodec);
if (!pCodecCtx) {
fprintf(stderr, "Failed to allocate codec context\n");
return -1;
}
if (avcodec_parameters_to_context(pCodecCtx, pCodecParams) < 0) {
fprintf(stderr, "Failed to copy codec parameters to codec context\n");
return -1;
}
if (avcodec_open2(pCodecCtx, pCodec, NULL) < 0) {
fprintf(stderr, "Failed to open codec\n");
return -1;
}
```
4. 创建AVPacket和AVFrame对象,循环读取并解码视频帧
```c
AVPacket *pPacket = av_packet_alloc();
if (!pPacket) {
fprintf(stderr, "Failed to allocate packet\n");
return -1;
}
AVFrame *pFrame = av_frame_alloc();
if (!pFrame) {
fprintf(stderr, "Failed to allocate frame\n");
return -1;
}
int ret;
while (av_read_frame(pFormatCtx, pPacket) >= 0) {
if (pPacket->stream_index == video_stream_idx) {
ret = avcodec_send_packet(pCodecCtx, pPacket);
if (ret < 0) {
fprintf(stderr, "Error sending a packet for decoding\n");
return -1;
}
while (ret >= 0) {
ret = avcodec_receive_frame(pCodecCtx, pFrame);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF) {
break;
} else if (ret < 0) {
fprintf(stderr, "Error during decoding\n");
return -1;
}
// Do something with the decoded frame here
}
}
av_packet_unref(pPacket);
}
```
5. 释放资源
```c
av_frame_free(&pFrame);
av_packet_free(&pPacket);
avcodec_free_context(&pCodecCtx);
avformat_close_input(&pFormatCtx);
```
以上代码是一个基本的解码H.265视频流的示例。在实际应用中,还需要根据具体需求进行适当的修改和优化。
阅读全文
相关推荐
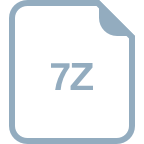
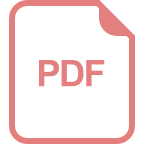
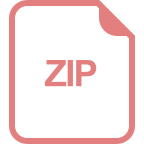
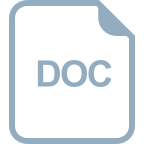
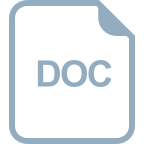
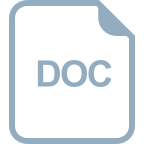
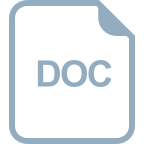
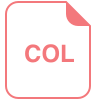
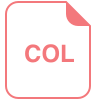
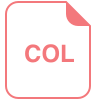
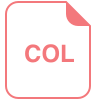
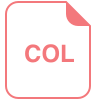



