生成一个python的推箱子游戏
时间: 2023-07-04 14:18:48 浏览: 43
好的,推箱子游戏是一种非常有趣的益智游戏,下面是一个简单的Python实现:
```python
# 地图中各个元素的表示
WALL = "#"
PLAYER = "P"
BOX = "B"
TARGET = "T"
FLOOR = " "
# 地图
MAP = [
"#####",
"# #",
"# B #",
"#PT #",
"#####"
]
# 初始化
def init():
global player_pos, boxes_pos, targets_pos, map_array
player_pos = None
boxes_pos = []
targets_pos = []
map_array = []
# 将地图转换为二维数组
for i, row in enumerate(MAP):
map_array.append([])
for j, col in enumerate(row):
map_array[i].append(col)
# 记录玩家和箱子的位置
if col == PLAYER:
player_pos = (i, j)
elif col == BOX:
boxes_pos.append((i, j))
elif col == TARGET:
targets_pos.append((i, j))
# 游戏是否结束
def is_game_over():
for box_pos in boxes_pos:
if box_pos not in targets_pos:
return False
return True
# 移动玩家或箱子
def move(pos, offset):
i, j = pos
x, y = offset
next_pos = (i + x, j + y)
# 判断下一个位置是否合法
if map_array[next_pos[0]][next_pos[1]] == WALL:
return False
# 如果下一个位置是箱子,移动箱子
if next_pos in boxes_pos:
if not move(next_pos, offset):
return False
# 移动玩家或箱子
map_array[i][j], map_array[next_pos[0]][next_pos[1]] = FLOOR, map_array[i][j]
pos = next_pos
# 更新玩家或箱子的位置
if pos == player_pos:
player_pos = next_pos
else:
boxes_pos[boxes_pos.index(pos)] = next_pos
return True
# 游戏主循环
def main_loop():
while not is_game_over():
# 打印地图
for row in map_array:
print("".join(row))
# 获取玩家输入
direction = input("请输入移动方向(WASD):").lower()
# 移动玩家
if direction == "w":
move(player_pos, (-1, 0))
elif direction == "s":
move(player_pos, (1, 0))
elif direction == "a":
move(player_pos, (0, -1))
elif direction == "d":
move(player_pos, (0, 1))
print("恭喜你,游戏结束!")
# 初始化游戏
init()
# 开始游戏
main_loop()
```
在这个游戏中,玩家需要控制一个角色推动箱子到指定位置。玩家输入WASD来移动角色,如果角色碰到了箱子,箱子也会跟着移动。当所有箱子都被推到指定位置时,游戏结束。
相关推荐
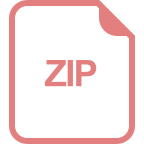
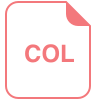
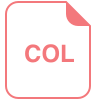
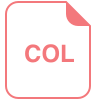

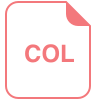








