package zzl3104.dongwu; abstract class Animal { private String name; public Animal(String name) { this.name = name; } public abstract void shout(); public abstract void eat(Food food); public String getName() { return name; } public void setName(String name) { this.name = name; } } class Dog extends Animal { public Dog(String name) { super(name); } @Override public void shout() { System.out.println("汪汪汪~~~"); } @Override public void eat(Food food) { System.out.println(getName() +"正在啃着香喷喷的" + food.getName()); } } class Cat extends Animal{ public Cat(String name) { super(name); } public void shout() { System.out.println("喵喵喵~~~"); } public void eat(Food food) { System.out.println(getName() + "正在吃着香喷喷的" + food.getName()); } } interface Food { public abstract String getName(); } class Bone implements Food { @Override public String getName() { return "骨头"; } } class Fish extends Animal implements Food { public Fish(String name) { super(name); } @Override public void shout() { } @Override public void eat(Food food) { } } public class Feeder { private String name; public Feeder(String name) { this.name = name; } public void speak() { System.out.println("欢迎来到动物园!"); System.out.println("我是饲养员" + getName()); } public void feed(Animal a, Food food) { a.eat(food); } public String getName() { return name; } public void setName(String name) { this.name = name; } } class DongWuTest { public static void main(String[] args) { Feeder feeder = new Feeder("小华"); feeder.speak(); Dog dog = new Dog("小狗"); dog.shout(); Food food = new Bone(); feeder.feed(dog, food); Cat cat = new Cat("小猫"); cat.shout(); food = new Fish("黄花鱼"); feeder.feed(cat, food); } }
时间: 2023-06-20 15:07:09 浏览: 247
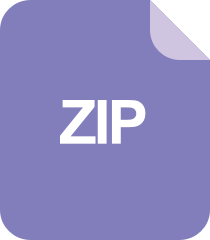
node本地服务端,可搭配花生壳的域名远程访问本地文件
这是一段Java代码,定义了一个抽象类Animal和其子类Dog和Cat,还有一个实现了Food接口的类Bone和一个既是Animal又是Food的类Fish。
Feeder类是饲养员类,可以说话,并能够喂养动物。在DongWuTest类中,实例化了一个Feeder对象,并通过它喂养了一只狗和一只猫,分别喂了骨头和黄花鱼。
阅读全文
相关推荐
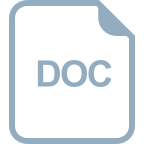
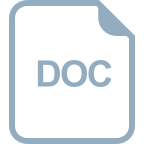
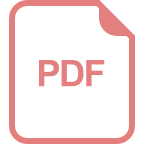
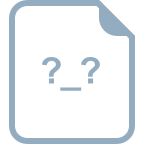










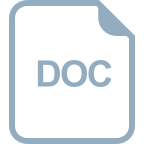
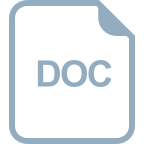