生产者消费者问题c实现
时间: 2024-09-15 22:09:39 浏览: 7
生产者消费者问题是计算机科学中经典的同步问题,也是操作系统课程中一个重要的概念。在该问题中,有一组生产者线程和一组消费者线程共享一个缓冲区。生产者线程向缓冲区中放入产品,而消费者线程则从缓冲区中取走产品。为了避免竞态条件(多个线程同时访问同一共享资源)和死锁问题,需要通过某种机制来同步这些线程的操作。下面是一种基于信号量的C语言实现方式。
```
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <semaphore.h>
#define BUFFER_SIZE 5
#define PRODUCER_NUM 3
#define CONSUMER_NUM 2
int buffer[BUFFER_SIZE]; // 缓冲区
int in = 0; // 缓冲区插入位置
int out = 0; // 缓冲区读取位置
sem_t empty; // 空槽数量
sem_t full; // 满槽数量
pthread_mutex_t mutex; // 互斥锁
void *producer(void *arg) {
int id = *(int*) arg;
while (1) {
int item = rand() % 100; // 生产随机整数
sem_wait(&empty); // 等待空槽
pthread_mutex_lock(&mutex); // 获取互斥锁
buffer[in] = item; // 插入数据
printf("Producer %d produced item %d at position %d.\n", id, item, in);
in = (in + 1) % BUFFER_SIZE; // 更新插入位置
pthread_mutex_unlock(&mutex); // 释放互斥锁
sem_post(&full); // 增加满槽数量
sleep(rand() % 3); // 随机休眠一段时间
}
}
void *consumer(void *arg) {
int id = *(int*) arg;
while (1) {
sem_wait(&full); // 等待满槽
pthread_mutex_lock(&mutex); // 获取互斥锁
int item = buffer[out]; // 取出数据
printf("Consumer %d consumed item %d from position %d.\n", id, item, out);
out = (out + 1) % BUFFER_SIZE; // 更新读取位置
pthread_mutex_unlock(&mutex); // 释放互斥锁
sem_post(&empty); // 增加空槽数量
sleep(rand() % 3); // 随机休眠一段时间
}
}
int main() {
pthread_t producer_threads[PRODUCER_NUM];
pthread_t consumer_threads[CONSUMER_NUM];
int producer_ids[PRODUCER_NUM];
int consumer_ids[CONSUMER_NUM];
sem_init(&empty, 0, BUFFER_SIZE); // 初始化空槽数量为缓冲区大小
sem_init(&full, 0, 0); // 初始化满槽数量为0
pthread_mutex_init(&mutex, NULL); // 初始化互斥锁
for (int i = 0; i < PRODUCER_NUM; i++) {
producer_ids[i] = i + 1;
pthread_create(&producer_threads[i], NULL, producer, &producer_ids[i]);
}
for (int i = 0; i < CONSUMER_NUM; i++) {
consumer_ids[i] = i + 1;
pthread_create(&consumer_threads[i], NULL, consumer, &consumer_ids[i]);
}
for (int i = 0; i < PRODUCER_NUM; i++) {
pthread_join(producer_threads[i], NULL);
}
for (int i = 0; i < CONSUMER_NUM; i++) {
pthread_join(consumer_threads[i], NULL);
}
sem_destroy(&empty); // 销毁信号量
sem_destroy(&full);
pthread_mutex_destroy(&mutex); // 销毁互斥锁
return 0;
}
```
相关推荐
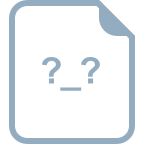
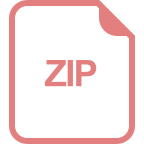
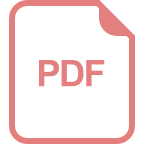














