promise封装ajax
时间: 2023-11-09 19:51:23 浏览: 78
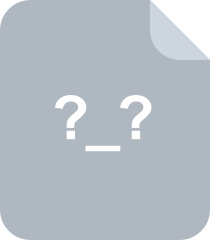
promise.js自定义封装,包含构造函数以及class封装
好的,以下是基于 Promise 封装的 AJAX 请求的示例代码:
```javascript
function ajaxRequest(url, method, data) {
// 创建 Promise 对象
return new Promise(function(resolve, reject) {
// 创建 XMLHttpRequest 对象
const xhr = new XMLHttpRequest();
// 设置请求的参数
xhr.open(method, url, true);
xhr.setRequestHeader('Content-Type', 'application/json');
// 监听请求状态的变化
xhr.onreadystatechange = function() {
if (xhr.readyState !== 4) {
return;
}
if (xhr.status >= 200 && xhr.status < 300) {
// 请求成功,解析响应数据并将其传递给 resolve 函数
resolve(JSON.parse(xhr.responseText));
} else {
// 请求失败,将错误信息传递给 reject 函数
reject(new Error(xhr.statusText));
}
};
// 发送请求
xhr.send(JSON.stringify(data));
});
}
```
使用示例:
```javascript
ajaxRequest('https://example.com/api', 'POST', { name: 'John', age: 30 })
.then(function(response) {
// 处理请求成功的响应数据
console.log(response);
})
.catch(function(error) {
// 处理请求失败的错误信息
console.error(error);
});
```
在上面的示例中,ajaxRequest 函数接受三个参数:请求的 URL、请求的方法(GET、POST 等)、请求的数据。它返回一个 Promise 对象,该对象在请求成功时会调用 resolve 函数,传递解析后的响应数据;在请求失败时会调用 reject 函数,传递一个 Error 对象,其中包含错误信息。
使用时,可以通过调用 then 方法来处理请求成功的响应数据,通过调用 catch 方法来处理请求失败的错误信息。
阅读全文
相关推荐


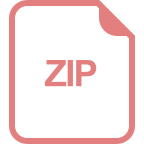
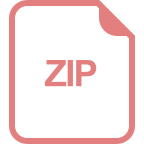
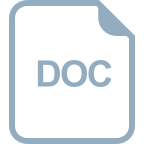






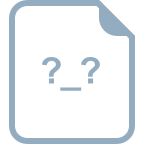
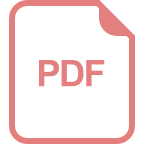
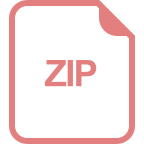