Modify the above code so that the positive and negative weights are random and the exact values are obtained from the objective function after Back propagation.
时间: 2024-04-08 18:29:05 浏览: 81
To modify the code so that the positive and negative weights are learned through backpropagation, you can define the weights as trainable parameters in the neural network class. Here's an updated version of the code:
```python
import torch
import torch.nn as nn
import torch.optim as optim
class RegressionNet(nn.Module):
def __init__(self, input_size, hidden_size):
super(RegressionNet, self).__init__()
# Positive sub-network
self.positive_net = nn.Sequential(
nn.Linear(input_size, hidden_size),
nn.ReLU(),
nn.Linear(hidden_size, 1)
)
# Negative sub-network
self.negative_net = nn.Sequential(
nn.Linear(input_size, hidden_size),
nn.ReLU(),
nn.Linear(hidden_size, 1)
)
# Initialize weights randomly
self.positive_weight = nn.Parameter(torch.randn(1))
self.negative_weight = nn.Parameter(torch.randn(1))
def forward(self, x):
positive_output = self.positive_weight * self.positive_net(x)
negative_output = -self.negative_weight * self.negative_net(x)
output = positive_output + negative_output
return output
# Example usage
input_size = 10
hidden_size = 20
model = RegressionNet(input_size, hidden_size)
# Generate dummy input data
batch_size = 32
input_data = torch.randn(batch_size, input_size)
target = torch.randn(batch_size, 1)
# Define loss function and optimizer
criterion = nn.MSELoss()
optimizer = optim.SGD(model.parameters(), lr=0.01)
# Training loop
num_epochs = 100
for epoch in range(num_epochs):
# Forward pass
output = model(input_data)
# Compute loss
loss = criterion(output, target)
# Backward pass and optimization
optimizer.zero_grad()
loss.backward()
optimizer.step()
# Print loss for monitoring
print(f"Epoch {epoch+1}/{num_epochs}, Loss: {loss.item()}")
# After training, you can access the learned weights
positive_weight = model.positive_weight.item()
negative_weight = model.negative_weight.item()
print(f"Positive weight: {positive_weight}, Negative weight: {negative_weight}")
```
In this updated code, we define `positive_weight` and `negative_weight` as trainable parameters using `nn.Parameter`. These parameters are initialized randomly and will be learned during the training process.
Inside the forward pass, we multiply the positive sub-network output by `positive_weight` and the negative sub-network output by `-negative_weight`. The rest of the code remains the same, with the addition of a training loop that performs forward and backward passes, updates the weights using an optimizer (here, stochastic gradient descent), and computes the loss for monitoring purposes.
After training, you can access the learned weights using `model.positive_weight.item()` and `model.negative_weight.item()`.
I hope this helps! Let me know if you have any further questions.
阅读全文
相关推荐
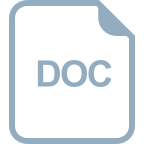
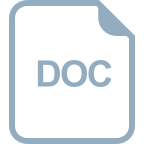
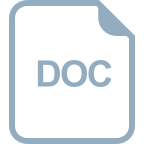


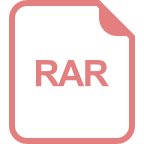
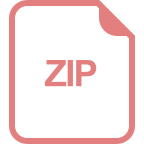
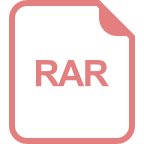
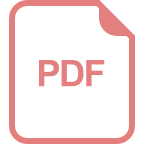
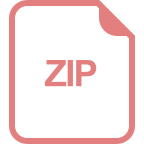
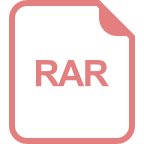
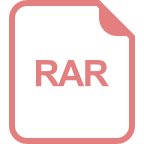
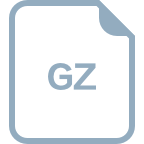
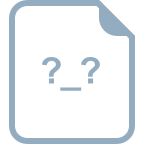
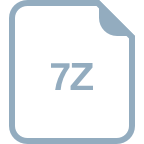
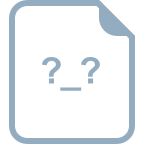