编写程序,给出一个字符串,将其中的字符"E”用空格替换后输出。
时间: 2023-09-06 12:04:37 浏览: 87
### 回答1:
可以使用字符串的 replace() 方法来实现:
```python
s = "Hello World"
s = s.replace("E", " ")
print(s)
```
输出结果为:
```
H llo World
```
### 回答2:
编写程序如下:
```python
string = input("请输入一个字符串:") # 输入一个字符串
new_string = string.replace("E", " ") # 将字符串中的"E"替换为空格
print("替换后的字符串为:", new_string) # 输出替换后的字符串
```
这段程序首先要求用户输入一个字符串,并将其存储在变量`string`中。然后,使用字符串的`replace()`方法将其中的字符"E"替换为空格,并将替换后的字符串存储在变量`new_string`中。最后,通过`print()`函数输出替换后的字符串。
注意:此程序示例使用的是Python编程语言,如果使用其他编程语言,请根据相应语言的语法和方法进行修改。
### 回答3:
编写程序可以使用循环遍历字符串的每个字符,如果当前字符为"E"则用空格替换,最后输出新的字符串。
```python
# 定义一个函数用于将字符串中的"E"替换为" "
def replace_E_with_space(string):
new_string = ""
for char in string:
if char == "E":
new_string += " "
else:
new_string += char
return new_string
# 测试示例
input_string = "Hello World!"
output_string = replace_E_with_space(input_string)
print(output_string)
```
以上代码中,replace_E_with_space函数遍历了输入的字符串,如果遇到字符"E"就将其替换为一个空格。最后将替换后的新字符串输出。
该程序的输出结果为:
```
H llo World!
```
> 注意:上述代码为Python的实现示例,因此要求在运行之前需要安装Python环境。如果你使用的是其他编程语言,可以根据相应语言的语法实现类似功能。
相关推荐
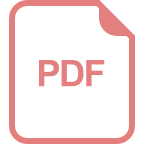
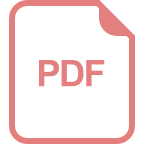
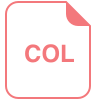
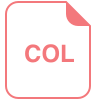
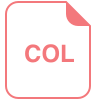
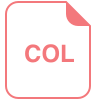
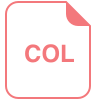









