用Python写一个爬虫,爬取双色球开奖记录
时间: 2023-02-22 18:09:24 浏览: 91
好的,下面是一个简单的爬虫代码,它爬取了双色球开奖记录:
```
import requests
from bs4 import BeautifulSoup
url = "http://kaijiang.500.com/ssq.shtml"
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
records = []
for tr in soup.find_all('tr')[2:]:
tds = tr.find_all('td')
date = tds[0].text
red_balls = [td.text for td in tds[2:8]]
blue_ball = tds[8].text
records.append({'date': date, 'red': red_balls, 'blue': blue_ball})
print(records)
```
这段代码使用了 `requests` 库爬取了网页内容,并使用 `BeautifulSoup` 库对网页内容进行解析。在解析后的网页内容中,通过遍历所有的 `<tr>` 标签,获取每次开奖的日期以及红球和蓝球的号码,最后将这些信息存入列表 `records` 中。
相关问题
用python写一个爬虫爬取音乐
### 回答1:
当然可以。爬取音乐数据有很多方法,具体实现方式可能会有所差别。下面是一个简单的例子,展示了如何使用 Python 爬取音乐数据:
首先,我们需要安装几个库,包括 Requests 和 BeautifulSoup。
```python
pip install requests
pip install beautifulsoup4
```
然后,我们可以使用 Requests 库来发送 HTTP 请求,获取网页内容。
```python
import requests
url = 'https://www.example.com/music'
response = requests.get(url)
html = response.text
```
接下来,我们可以使用 BeautifulSoup 解析 HTML 网页,提取出我们想要的信息。
```python
from bs4 import BeautifulSoup
soup = BeautifulSoup(html, 'html.parser')
# 提取音乐名称
music_names = soup.find_all('h2', class_='music-name')
for name in music_names:
print(name.text)
```
这是一个非常简单的爬虫示例,但是可以作为你开始爬取音乐数据的基础。你可以根据自己的需要来修改爬虫的代码,提取更多的信息。
希望这些信息能帮到你。
### 回答2:
使用Python编写爬虫来爬取音乐是可行的。以下是一个基本的爬虫示例,用于从一个音乐网站上获取音乐信息:
首先,我们需要导入必要的库,如requests和BeautifulSoup:
```python
import requests
from bs4 import BeautifulSoup
```
然后,我们可以定义一个函数来进行爬取音乐的操作。在这个函数中,我们可以使用requests库发送HTTP请求,获取网页的内容。然后,使用BeautifulSoup库对网页内容进行解析,提取出我们需要的音乐信息。
```python
def crawl_music():
url = "这里填入音乐网站的URL"
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
# 在这里使用soup对象的方法提取音乐信息,如歌曲名称、歌手等
# 例如,假设歌曲名称所在的HTML标签是<div class="song">,可以通过以下方式提取出所有的歌曲名称:
song_names = soup.find_all('div', class_='song')
for song_name in song_names:
print(song_name.text)
```
最后,我们可以调用这个函数来启动爬取过程:
```python
if __name__ == "__main__":
crawl_music()
```
请注意,这只是一个简单的示例,实际中可能需要根据具体的音乐网站进行相应的调整。同时,也要注意遵守相关的法律法规,确保合法使用爬虫程序。
### 回答3:
使用Python编写爬虫来爬取音乐可以通过以下步骤实现:
1. 导入所需的库:首先导入`requests`库用于向网页发送HTTP请求,以及`beautifulsoup4`库用于解析网页内容。
2. 发送HTTP请求获取网页内容:使用`requests`库发送GET请求获取音乐网站的网页内容,并将其保存在一个变量中。
3. 解析网页内容:使用`beautifulsoup4`库解析上一步获取的网页内容,以便提取所需的音乐信息。
4. 提取音乐信息:根据音乐网站的HTML结构,使用`beautifulsoup4`库提取出所需的音乐信息,例如音乐名称、URL链接等。
5. 下载音乐文件:使用`requests`库发送GET请求下载音乐文件,并保存到指定的文件夹中。
以下是一个简单的示例代码:
```
import requests
from bs4 import BeautifulSoup
# 发送HTTP请求获取网页内容
url = "https://music.com" # 替换为实际的音乐网站URL
response = requests.get(url)
content = response.content
# 解析网页内容
soup = BeautifulSoup(content, "html.parser")
# 提取音乐信息
music_list = soup.find_all("div", class_="music-item") # 根据实际的网页结构提取音乐信息
# 下载音乐文件
for music in music_list:
name = music.find("span", class_="music-name").text # 根据实际的网页结构提取音乐名称
music_url = music.find("a")["href"] # 根据实际的网页结构提取音乐链接
response = requests.get(music_url)
with open(f"{name}.mp3", "wb") as file: # 保存音乐文件到指定的文件夹
file.write(response.content)
```
请注意,以上代码只是一个简单的示例,实际应用中可能需要根据音乐网站的具体结构进行适当的修改和调整。此外,实际的爬虫开发中还需要考虑反爬机制、请求频率控制等其他因素。
用python写一个爬虫爬取指定电影
可以使用Python的第三方库如BeautifulSoup或Scrapy来编写一个爬虫。
首先,需要导入所需的库:
```
from bs4 import BeautifulSoup
import requests
```
然后,可以使用`requests`库发送HTTP请求获取目标网页的HTML代码:
```
url = "https://example.com/movies/target_movie"
response = requests.get(url)
html_content = response.text
```
接着,使用`BeautifulSoup`解析HTML代码并提取所需的信息:
```
soup = BeautifulSoup(html_content, 'html.parser')
title = soup.find('h1').text
year = soup.find('span', {'class': 'release-year'}).text
```
最后,将提取的信息打印出来:
```
print("电影名称:", title)
print("上映年份:", year)
```
需要注意的是,爬虫需要遵循网站的使用条款和隐私政策,并且在爬取数据时需要考虑到效率和反爬虫措施。
相关推荐
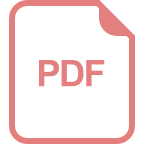
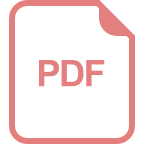












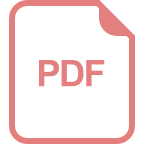