点击按钮在Android Studio中进行开发任务时,如何将sqlite数据库中的内容去显示在应用界面全部代码
时间: 2023-08-18 20:08:52 浏览: 113
首先,你需要在你的应用中创建一个SQLite数据库并将数据插入到其中。然后,你需要在应用中创建一个布局以显示数据库中的数据。最后,你需要编写代码来检索数据库中的数据并将其显示在应用中。
下面是一个简单的示例,演示如何在Android应用中显示SQLite数据库中的数据。假设你的数据库中有一个名为“people”的表,其中包含“name”和“age”两个列。
在你的应用中,你需要创建一个布局文件来显示数据库中的数据。以下是一个简单的布局文件示例:
```xml
<LinearLayout
android:id="@+id/layout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/title"
android:text="People"
android:textSize="24sp"
android:layout_marginTop="16dp"
android:layout_marginBottom="16dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<ListView
android:id="@+id/listview"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
</LinearLayout>
```
接下来,你需要编写一个类来检索数据库中的数据并将其显示在ListView中。以下是一个简单的示例:
```java
public class MainActivity extends AppCompatActivity {
private ListView listView;
private List<Person> peopleList;
private PersonAdapter adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
listView = findViewById(R.id.listview);
peopleList = new ArrayList<>();
adapter = new PersonAdapter(this, peopleList);
listView.setAdapter(adapter);
// Retrieve data from database
SQLiteDatabase db = openOrCreateDatabase("mydatabase", MODE_PRIVATE, null);
Cursor cursor = db.rawQuery("SELECT * FROM people", null);
// Add data to list
if (cursor.moveToFirst()) {
do {
String name = cursor.getString(cursor.getColumnIndex("name"));
int age = cursor.getInt(cursor.getColumnIndex("age"));
Person person = new Person(name, age);
peopleList.add(person);
} while (cursor.moveToNext());
}
// Update adapter
adapter.notifyDataSetChanged();
// Close database and cursor
cursor.close();
db.close();
}
}
```
在上面的代码中,我们首先获取ListView并创建一个空的人员列表和一个自定义的PersonAdapter。然后我们打开数据库并检索所有人员的姓名和年龄。我们将这些数据添加到人员列表中,并通知适配器数据已更改。最后,我们关闭数据库和游标。
以下是自定义Person类和PersonAdapter类的示例:
```java
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
public class PersonAdapter extends ArrayAdapter<Person> {
public PersonAdapter(Context context, List<Person> people) {
super(context, 0, people);
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
if (convertView == null) {
convertView = LayoutInflater.from(getContext()).inflate(android.R.layout.simple_list_item_2, parent, false);
}
Person person = getItem(position);
TextView nameView = convertView.findViewById(android.R.id.text1);
nameView.setText(person.getName());
TextView ageView = convertView.findViewById(android.R.id.text2);
ageView.setText(String.valueOf(person.getAge()));
return convertView;
}
}
```
在上面的代码中,我们创建了一个Person类来表示人员,并创建了一个PersonAdapter类来将人员列表显示在ListView中。在getView方法中,我们使用Android提供的简单列表项布局来显示每个人员的姓名和年龄。
希望这个示例可以帮助你开始在你的Android应用中显示SQLite数据库中的数据!
相关推荐
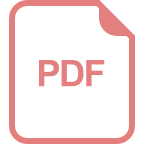
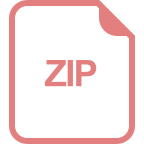














