C语言strstr返回值
时间: 2024-08-02 14:01:17 浏览: 203
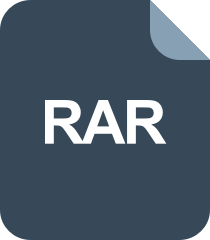
数据预处理C语言.rar
`strstr()`是C语言标准库函数,它用于在一个字符串中查找指定子串首次出现的位置。如果找到子串,则返回指向该子串的首字符的指针;如果没有找到,则返回`NULL`。这个函数常用于字符串操作,特别是当需要判断某个子串是否存在于另一个字符串中。
例如:
```c
char *str = "Hello, World!";
char *substr = "World";
char *result = strstr(str, substr);
if (result) {
printf("Substring found at position %ld\n", result - str); // 输出: Substring found at position 7
} else {
printf("Substring not found.\n");
}
```
在这里,`strstr(str, substr)`会返回`"World"`的第一个字符的地址(即`&str`),因为子串从索引0开始算,所以地址减去原始字符串的地址就是起始位置。
阅读全文
相关推荐
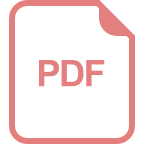
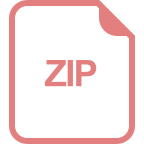






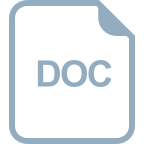





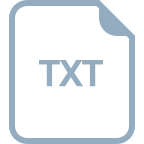


