计算根据某点坐标图片缩放偏移量
时间: 2023-09-10 16:07:43 浏览: 274
计算根据某点坐标图片缩放偏移量的公式如下:
假设图片原始大小为 `width x height`,缩放后的大小为 `newWidth x newHeight`,某点的坐标为 `(pointX, pointY)`,缩放比例为 `scale`。
则,缩放偏移量的坐标可以通过以下公式计算得出:
```
offsetX = pointX - (pointX / scale)
offsetY = pointY - (pointY / scale)
```
其中,`(pointX / scale)` 和 `(pointY / scale)` 分别表示缩放后的坐标,`pointX` 和 `pointY` 分别表示缩放前的坐标。这个公式的基本思想是:先将缩放前的坐标转换成缩放后的坐标,然后计算出偏移量,最终得到缩放偏移量的坐标。
相关问题
// 鼠标按下事件 imgContainer.onmousedown = function (e) { e.preventDefault(); isDragging = true; startX = e.clientX; startY = e.clientY; startScrollLeft = imgContainer.scrollLeft; startScrollTop = imgContainer.scrollTop; }; // 鼠标移动事件 imgContainer.onmousemove = function (e) { if (isDragging) { var moveX = e.clientX - startX; var moveY = e.clientY - startY; imgContainer.scrollLeft = startScrollLeft - moveX; imgContainer.scrollTop = startScrollTop - moveY; } }; // 鼠标释放事件 imgContainer.onmouseup = function (e) { isDragging = false; }; // 鼠标离开事件 imgContainer.onmouseleave = function (e) { isDragging = false; }; // 鼠标滚轮事件 img.onwheel = function (e) { e.preventDefault(); // 阻止默认滚轮行为处理页面滚动 var rect = img.getBoundingClientRect(); var mouseX = e.clientX - rect.left; // 鼠标在图片上的相对X坐标 var mouseY = e.clientY - rect.top; // 鼠标在图片上的相对Y坐标 var oldWidth = rect.width; // 原始图片宽度 var oldHeight = rect.height; // 原始图片高度 scale += e.deltaY > 0 ? -scaleFactor : scaleFactor; // 根据滚轮滚动方向确定缩放比例 scale = Math.max(scale, 0.1); // 最小缩放比例为0.1 var newWidth = oldWidth * scale; // 缩放后的宽度 var newHeight = oldHeight * scale; // 缩放后的高度 var scaleX = newWidth / oldWidth; // 宽度缩放比例 var scaleY = newHeight / oldHeight; // 高度缩放比例 var offsetX = (mouseX - rect.width / 2) * scaleX; // X轴偏移量 var offsetY = (mouseY - rect.height / 2) * scaleY; // Y轴偏移量 img.style.transform = scale(${scale}) translate(${offsetX}px, ${offsetY}px); }; // 关闭悬浮窗 popup.onclick = function () { document.body.removeChild(popup); isPopupVisible = false; // 标记悬浮窗为隐藏状态 }; imgContainer.appendChild(img); popup.appendChild(imgContainer); document.body.appendChild(popup); }这是修改后代码,任然存在图片跳动,无法正常放大问题
在代码中,图片跳动的问题可能是由于鼠标滚轮事件处理函数中的缩放和偏移计算不准确导致的。你可以尝试修改代码,将缩放和偏移计算的逻辑分开处理,以确保计算的准确性。修改后的代码如下:
```javascript
// 鼠标滚轮事件
img.onwheel = function (e) {
e.preventDefault(); // 阻止默认滚轮行为处理页面滚动
var rect = img.getBoundingClientRect();
var mouseX = e.clientX - rect.left; // 鼠标在图片上的相对X坐标
var mouseY = e.clientY - rect.top; // 鼠标在图片上的相对Y坐标
var oldWidth = rect.width; // 原始图片宽度
var oldHeight = rect.height; // 原始图片高度
scale += e.deltaY > 0 ? -scaleFactor : scaleFactor; // 根据滚轮滚动方向确定缩放比例
scale = Math.max(scale, 0.1); // 最小缩放比例为0.1
var newWidth = oldWidth * scale; // 缩放后的宽度
var newHeight = oldHeight * scale; // 缩放后的高度
var scaleX = newWidth / oldWidth; // 宽度缩放比例
var scaleY = newHeight / oldHeight; // 高度缩放比例
var offsetX = (mouseX - rect.width / 2) * scaleX; // X轴偏移量
var offsetY = (mouseY - rect.height / 2) * scaleY; // Y轴偏移量
var newOffsetX = offsetX - (newWidth - oldWidth) / 2; // 考虑缩放引起的偏移变化
var newOffsetY = offsetY - (newHeight - oldHeight) / 2; // 考虑缩放引起的偏移变化
img.style.transform = `scale(${scale}) translate(${newOffsetX}px, ${newOffsetY}px)`;
};
```
请尝试使用上述修改后的代码,看看是否能够解决图片跳动的问题。如果问题仍然存在,请提供更多相关代码或详细描述你想要实现的效果,以便我能够提供更准确的帮助。
以鼠标为中心点,transform进行图片缩放的JavaScript代码
以下是以鼠标为中心点进行图片缩放的JavaScript代码:
```javascript
// 获取图片对象
var img = document.getElementById("myImage");
// 监听鼠标滚轮事件
img.addEventListener("wheel", function(e) {
// 阻止默认事件,避免页面滚动
e.preventDefault();
// 计算缩放比例
var scale = e.deltaY > 0 ? 0.9 : 1.1;
// 获取鼠标相对于图片左上角的坐标
var x = e.clientX - img.offsetLeft;
var y = e.clientY - img.offsetTop;
// 计算缩放后的坐标
var newX = x * scale;
var newY = y * scale;
// 计算坐标的偏移量
var deltaX = newX - x;
var deltaY = newY - y;
// 设置 transform 属性
img.style.transformOrigin = deltaX + "px " + deltaY + "px";
img.style.transform = "scale(" + scale + ")";
});
```
其中,`myImage` 是图片的 id,可以根据实际情况进行修改。这段代码通过监听鼠标滚轮事件,获取鼠标相对于图片左上角的坐标,并根据鼠标滚动方向计算缩放比例,最后设置 transform 属性实现图片缩放。
阅读全文
相关推荐
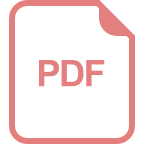
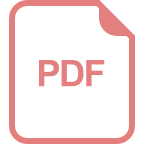
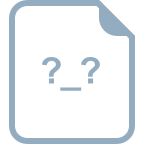
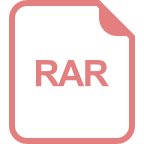
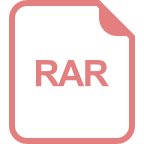
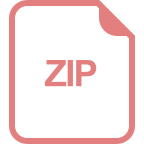
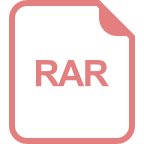
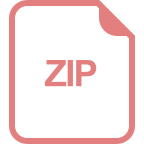
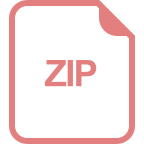
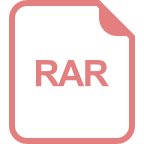
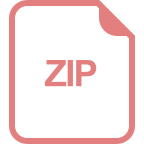
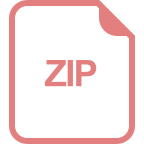
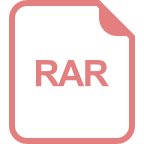
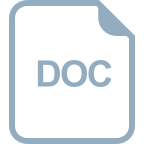
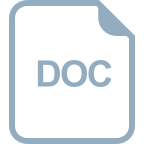
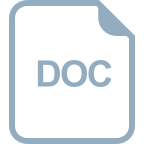