python连接mysql数据库详细方法及步骤
时间: 2023-07-28 16:10:29 浏览: 139
连接MySQL数据库的详细方法及步骤如下:
1. 安装MySQL驱动程序
在Python中连接MySQL数据库需要使用MySQL驱动程序,我们可以使用pip命令安装PyMySQL或mysql-connector-python。
- 使用PyMySQL:
```python
pip install PyMySQL
```
- 使用mysql-connector-python:
```python
pip install mysql-connector-python
```
2. 导入MySQL驱动程序
在Python代码中导入MySQL驱动程序,如果使用PyMySQL,则导入PyMySQL模块,如果使用mysql-connector-python,则导入mysql.connector模块。
```python
# 使用PyMySQL
import pymysql
# 使用mysql-connector-python
import mysql.connector
```
3. 连接MySQL数据库
在Python代码中连接MySQL数据库,需要指定数据库的主机、用户名、密码、数据库名称等信息。
- 使用PyMySQL:
```python
# 打开数据库连接
db = pymysql.connect(host="localhost", user="root", password="password", database="test")
# 关闭数据库连接
db.close()
```
- 使用mysql-connector-python:
```python
# 打开数据库连接
db = mysql.connector.connect(host="localhost", user="root", password="password", database="test")
# 关闭数据库连接
db.close()
```
4. 创建游标对象
在Python代码中连接MySQL数据库后,需要创建一个游标对象,用于执行SQL语句。
- 使用PyMySQL:
```python
# 创建游标对象
cursor = db.cursor()
# 关闭游标对象
cursor.close()
```
- 使用mysql-connector-python:
```python
# 创建游标对象
cursor = db.cursor()
# 关闭游标对象
cursor.close()
```
5. 执行SQL语句
在Python代码中连接MySQL数据库后,使用游标对象执行SQL语句。
- 使用PyMySQL:
```python
# 执行SQL语句
sql = "SELECT * FROM table_name"
cursor.execute(sql)
# 获取查询结果
results = cursor.fetchall()
for row in results:
print(row)
# 提交修改
db.commit()
```
- 使用mysql-connector-python:
```python
# 执行SQL语句
sql = "SELECT * FROM table_name"
cursor.execute(sql)
# 获取查询结果
results = cursor.fetchall()
for row in results:
print(row)
# 提交修改
db.commit()
```
6. 关闭数据库连接
在Python代码中连接MySQL数据库后,需要在完成操作后关闭数据库连接。
```python
# 关闭数据库连接
db.close()
```
以上就是Python连接MySQL数据库的详细方法及步骤。
阅读全文
相关推荐

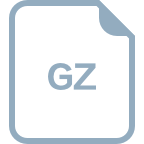












