用python编程凯撒密码
时间: 2024-07-02 19:01:22 浏览: 136
凯撒密码(Caesar Cipher)是一种简单的替换式加密技术,最早由古罗马时期的凯撒大帝使用。在Python中,我们可以轻松地编写一个简单的程序来实现凯撒密码。凯撒密码的基本思想是将明文中的每个字母按照固定的位移(比如3位)向后(或向前)移动。
以下是一个简单的Python代码示例,用于加密和解密凯撒密码:
```python
def caesar_cipher_encrypt(text, shift):
encrypted_text = ""
for char in text:
if char.isalpha():
shift_amount = shift % 26 # 防止字母超出A-Z或a-z范围
if char.islower():
encrypted_text += chr((ord(char) - ord('a') + shift_amount) % 26 + ord('a'))
else:
encrypted_text += chr((ord(char) - ord('A') + shift_amount) % 26 + ord('A'))
else:
encrypted_text += char # 保留非字母字符不变
return encrypted_text
def caesar_cipher_decrypt(encrypted_text, shift):
return caesar_cipher_encrypt(encrypted_text, -shift)
# 示例
plaintext = "Hello, World!"
shift = 3
encrypted_text = caesar_cipher_encrypt(plaintext, shift)
print(f"Encrypted text: {encrypted_text}")
decrypted_text = caesar_cipher_decrypt(encrypted_text, shift)
print(f"Decrypted text: {decrypted_text}")
```
阅读全文
相关推荐
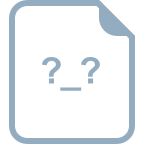
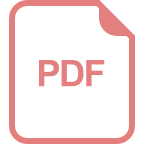
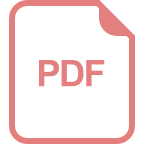
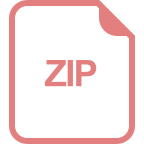
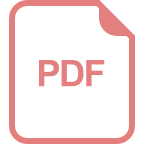
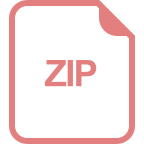
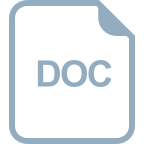
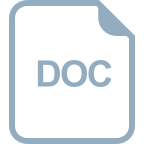
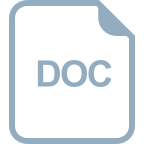
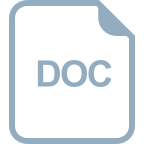
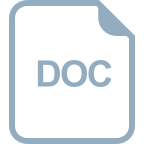







