用c实现两个有序链表序列的合并
时间: 2024-05-02 11:21:48 浏览: 18
以下是C语言实现两个有序链表序列合并的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
struct Node* mergeLists(struct Node* head1, struct Node* head2) {
struct Node* mergedList = NULL;
struct Node** tailRef = &mergedList;
while (1) {
if (head1 == NULL) {
*tailRef = head2;
break;
} else if (head2 == NULL) {
*tailRef = head1;
break;
}
if (head1->data <= head2->data) {
*tailRef = head1;
head1 = head1->next;
} else {
*tailRef = head2;
head2 = head2->next;
}
tailRef = &((*tailRef)->next);
}
return mergedList;
}
void printList(struct Node* head) {
while (head != NULL) {
printf("%d ", head->data);
head = head->next;
}
printf("\n");
}
int main() {
struct Node* head1 = NULL;
struct Node* head2 = NULL;
struct Node* mergedList = NULL;
// create first list
head1 = (struct Node*)malloc(sizeof(struct Node));
head1->data = 1;
head1->next = (struct Node*)malloc(sizeof(struct Node));
head1->next->data = 3;
head1->next->next = (struct Node*)malloc(sizeof(struct Node));
head1->next->next->data = 5;
head1->next->next->next = NULL;
// create second list
head2 = (struct Node*)malloc(sizeof(struct Node));
head2->data = 2;
head2->next = (struct Node*)malloc(sizeof(struct Node));
head2->next->data = 4;
head2->next->next = (struct Node*)malloc(sizeof(struct Node));
head2->next->next->data = 6;
head2->next->next->next = NULL;
printf("List 1: ");
printList(head1);
printf("List 2: ");
printList(head2);
mergedList = mergeLists(head1, head2);
printf("Merged list: ");
printList(mergedList);
return 0;
}
```
该程序首先定义了一个结构体`Node`,表示链表的节点。`mergeLists()`函数接受两个有序链表的头结点作为参数,然后遍历这两个链表并将它们合并成一个有序链表。该函数使用双重指针来维护新链表的尾节点,以便可以将新节点添加到新链表的末尾。最后,该函数返回已合并的有序链表的头结点。
`printList()`函数用于打印链表中所有节点的值。在`main()`函数中,创建两个有序链表,然后调用`mergeLists()`函数将它们合并成一个新链表,并打印新链表的所有节点的值。
相关推荐
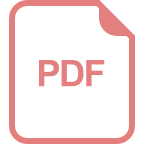
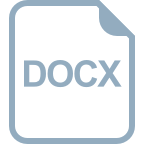














