C# UIAutomation获取某个程序的截图
时间: 2024-10-07 21:02:00 浏览: 44
在C#中,如果你想要通过UI Automation获取特定应用程序的屏幕截图,你可以使用`System.Drawing`库中的`Bitmap`类配合Windows API函数来实现。以下是一个简单的步骤:
1. 首先,需要引用`System.Drawing`和`System.Runtime.InteropServices`命名空间。
```csharp
using System.Drawing;
using System.Runtime.InteropServices;
```
2. 使用`User32.dll`库中的`GetWindowDC`和`BitBlt`函数获取窗口的设备上下文(Device Context),然后创建一个新的`Bitmap`对象。
```csharp
[DllImport("user32.dll", SetLastError = true)]
static extern IntPtr GetWindowDC(IntPtr hWnd);
[DllImport("gdi32.dll")]
static extern bool BitBlt(IntPtr h_dstDC, int xDest, int yDest,
int nWidth, int nHeight, IntPtr h_srcDC, int xSrc, int ySrc,
CopyPixelOperation op);
```
3. 获取目标应用程序的主窗口句柄(`hWnd`)。这通常可以通过UI Automation找到,例如使用`FindWindow`函数。假设已经有一个名为`targetAppName`的字符串,表示你要截图的应用程序名称。
```csharp
IntPtr hWnd = FindWindow(null, targetAppName); // 替换为目标应用程序的名称
if (hWnd == IntPtr.Zero)
{
throw new Exception($"未能找到窗口:{targetAppName}");
}
```
4. 获取窗口设备上下文(DC)并复制到一个内存DC。
```csharp
IntPtr hdcWindow = GetWindowDC(hWnd);
try
{
IntPtr hdcMemory = CreateCompatibleDC(hdcWindow);
try
{
Bitmap bitmap = new Bitmap(nWidth, nHeight, PixelFormat.Format32bppArgb);
IntPtr hdcBitmap = bitmap.GetHdc();
try
{
BitBlt(hdcBitmap, 0, 0, bitmap.Width, bitmap.Height, hdcWindow, 0, 0, CopyPixelOperation.SourceCopy);
// 现在可以保存或操作bitmap
}
finally
{
bitmap.ReleaseHdc(hdcBitmap);
}
}
finally
{
DeleteDC(hdcMemory);
}
}
finally
{
ReleaseDC(hWnd, hdcWindow);
}
```
5. 最后,你可以将`Bitmap`转换为其他格式的图像文件(如JPG、PNG等)并保存,或者直接操作其像素数据。
```csharp
// 将Bitmap保存为JPEG图片
bitmap.Save("screenshot.jpg");
```
请注意,这个例子基于对窗口的直接操作,并非UI Automation,因为UI Automation通常用于自动化用户界面操作而不是截屏。如果你想通过UI Automation获取UI元素的截图,你应该使用UI Automation提供的API,如`CaptureElement()`方法。
阅读全文
相关推荐
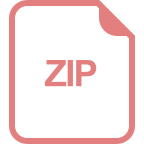
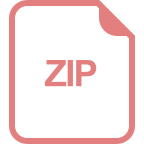
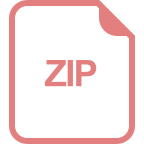

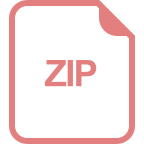


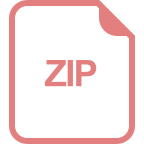
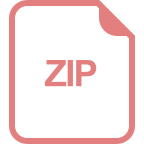





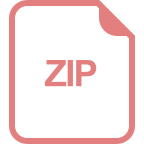
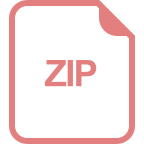
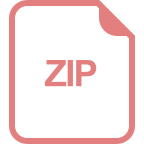