使用C#写一个解析A2L文件的详细代码并加上详细注释
时间: 2024-01-22 12:20:20 浏览: 40
以下是一个使用C#解析A2L文件的示例代码,包含详细注释:
```csharp
using System;
using System.IO;
using System.Text.RegularExpressions;
using System.Collections.Generic;
class A2LParser
{
static void Main(string[] args)
{
// 读取A2L文件
string a2lFilePath = "test.a2l";
string a2lContent = File.ReadAllText(a2lFilePath);
// 解析A2L文件
Dictionary<string, string> characteristics = ParseA2L(a2lContent);
// 打印解析结果
foreach (KeyValuePair<string, string> characteristic in characteristics)
{
Console.WriteLine(characteristic.Key + " = " + characteristic.Value);
}
}
static Dictionary<string, string> ParseA2L(string a2lContent)
{
// 创建一个字典,用于存储解析结果
Dictionary<string, string> characteristics = new Dictionary<string, string>();
// 定义正则表达式模式,用于匹配A2L文件中的特性定义
string regexPattern = @"(?<=CHARACTERISTIC\s+\")(.*?)(?=\"")\s+\""(.*?)\""";
// 使用正则表达式匹配特性定义,并将结果添加到字典中
foreach (Match match in Regex.Matches(a2lContent, regexPattern))
{
string characteristicName = match.Groups[1].Value;
string characteristicValue = match.Groups[2].Value;
characteristics.Add(characteristicName, characteristicValue);
}
return characteristics;
}
}
```
该代码使用正则表达式匹配A2L文件中的特性定义,并将结果存储到一个字典中。特性定义的格式如下:
```
CHARACTERISTIC "CharacteristicName" "CharacteristicValue"
```
其中,`CharacteristicName` 是特性的名称,`CharacteristicValue` 是特性的值。
在解析A2L文件时,该代码使用一个正则表达式模式来匹配特性定义,并将匹配结果添加到字典中。具体来说,代码中的 `regexPattern` 变量定义了一个正则表达式模式,用于匹配特性定义。该模式包含两个捕获组,分别用于匹配特性名称和特性值。
然后,代码使用 `Regex.Matches()` 方法来查找输入字符串中与模式匹配的所有字符串。对于每个匹配项,代码从捕获组中提取特性名称和特性值,并将它们添加到字典中。
最后,代码返回包含解析结果的字典。对于每个特性,字典中的键是特性名称,值是特性值。在主函数中,代码打印了解析结果。
相关推荐
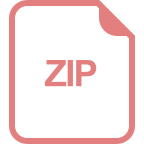














