auto作为函数形参
时间: 2023-11-11 20:15:11 浏览: 47
在 C++11 之前,函数的形参必须显式地指定类型,但是在 C++11 中,auto 关键字可以用于函数形参类型的推断。这种用法被称为 "函数模板自动类型推导"。
使用 auto 作为函数形参类型时,函数模板可以接受任意类型的参数,然后在函数体中使用 auto 来推导参数的类型。例如:
```
template<typename T>
void foo(T x)
{
// 在函数体中可以使用 auto 推导 x 的类型
auto y = x;
// ...
}
```
在上面的例子中,我们使用 auto 来推导函数参数 x 的类型。这样,函数 foo 就可以接受任意类型的参数。在函数体中,我们可以使用 auto 来推导 x 的类型,然后使用 y 来存储推导出来的类型。这种用法可以让代码更加简洁,并且可以避免显式地指定参数类型的繁琐。需要注意的是,使用 auto 作为函数形参类型时,应该避免过度使用,保持代码的可读性。
相关问题
auto推导函数指针
auto推导函数指针可以通过使用decltype关键字来完成。例如,假设有一个函数指针如下:
```cpp
int foo(int a, int b) {
return a + b;
}
int main() {
auto func_ptr = &foo;
return 0;
}
```
在这个例子中,auto关键字将自动推导func_ptr的类型为int (*)(int, int),即指向接受两个int参数并返回int的函数的指针。
autolisp函数参考手册下载
Autolisp是一种用于自动化CAD绘图的编程语言。它可以通过编写程序来实现CAD的自动化绘图、自定义命令及自动计算等功能。而Autolisp函数是Autolisp语言的核心,函数定义了Autolisp程序中的操作和处理方式。
了解Autolisp函数的使用方法对于编写Autolisp程序必不可少。因此,Autolisp函数参考手册是Autolisp程序员所必需的工具。Autolisp函数参考手册是一份详细的文档,其中列举了Autolisp中所有可用的函数及其参数、返回值等信息。程序员可以通过手册检索自己需要的函数,了解函数的使用方法、参数要求及返回结果等信息,以便编写Autolisp程序。
Autolisp函数参考手册是免费提供的,任何人都可以通过官方网站进行下载,网站为:www.autodesk.com。手册中包含了Autolisp的核心函数库,包括三维绘图、Entity操作、用户界面、文件操作、表格处理等方面的函数。在使用过程中,程序员可以根据需要选择合适的函数,并结合自己的需要进行自由应用。
总之,Autolisp函数参考手册是Autolisp程序员不可或缺的工具。通过手册的使用,程序员能够更好地了解Autolisp语言的核心,熟练掌握Autolisp编程基础知识,编写高效的Autolisp程序,提高CAD绘图效率。
相关推荐
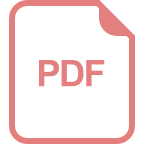
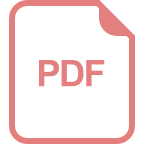
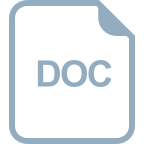












