robocode坦克代码
时间: 2023-10-20 12:08:00 浏览: 99
以下是一个简单的 Robocode 坦克代码示例:
```java
import robocode.*;
public class MyFirstRobot extends Robot {
public void run() {
while (true) {
ahead(100); // 向前移动 100 个像素
turnGunRight(360); // 旋转炮塔一圈
back(100); // 向后移动 100 个像素
turnGunRight(360); // 旋转炮塔一圈
}
}
public void onScannedRobot(ScannedRobotEvent e) {
fire(1); // 发射一枚火箭
}
public void onHitByBullet(HitByBulletEvent e) {
back(50); // 被子弹击中后向后移动 50 个像素
}
}
```
这个坦克会不断地前进、旋转炮塔并向后移动,同时如果发现敌方坦克则会开火,被子弹击中后会退后。你可以根据自己的需要进行修改和扩展。
相关问题
robocode强化坦克代码
以下是一个稍微复杂一些的 Robocode 强化坦克代码示例:
```java
import robocode.*;
public class MyStrongRobot extends AdvancedRobot {
double previousEnergy = 100;
int moveDirection = 1;
public void run() {
setAdjustGunForRobotTurn(true);
setAdjustRadarForGunTurn(true);
turnRadarRightRadians(Double.POSITIVE_INFINITY); // 扫描整个场地
while (true) {
turnRight(5 * moveDirection); // 每次转动 5 度
if (getEnergy() < 50) { // 当能量低于 50 时
moveDirection = -moveDirection; // 反向移动
setAhead(100 * moveDirection); // 移动 100 个像素
}
execute(); // 执行命令
}
}
public void onScannedRobot(ScannedRobotEvent e) {
double bearing = getHeadingRadians() + e.getBearingRadians();
double gunTurn = bearing - getGunHeadingRadians();
double radarTurn = bearing - getRadarHeadingRadians() + Math.PI / 2;
setTurnGunRightRadians(gunTurn);
setTurnRadarRightRadians(radarTurn);
if (getEnergy() > 50) { // 当能量高于 50 时
fire(1 + getEnergy() / 50); // 根据能量大小调整火力
} else {
fire(1); // 火力为 1
}
if (e.getEnergy() < previousEnergy) { // 当敌方坦克掉血时
moveDirection = -moveDirection; // 反向移动
setAhead(100 * moveDirection); // 移动 100 个像素
}
previousEnergy = e.getEnergy(); // 更新敌方坦克的血量
}
public void onHitByBullet(HitByBulletEvent e) {
moveDirection = -moveDirection; // 反向移动
setAhead(100 * moveDirection); // 移动 100 个像素
}
}
```
这个坦克会不断地转动炮塔和雷达,并根据敌方坦克的位置和能量大小调整炮塔的方向和火力。当自己的能量低于 50 时,会改变移动方向并向前移动 100 个像素。如果被子弹击中,则会反向移动 100 个像素。这个坦克还会通过比较敌方坦克的血量前后变化来判断敌方坦克是否掉血,从而调整自己的移动方向。你可以根据自己的需要进行修改和扩展。
阅读全文
相关推荐
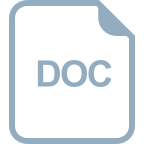
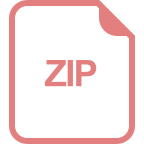
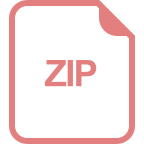
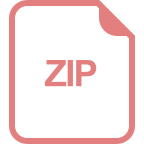
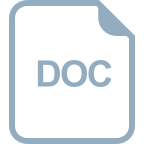
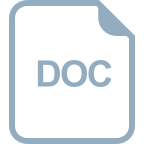
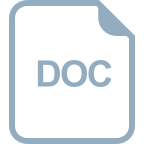
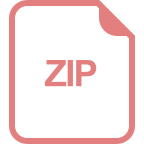
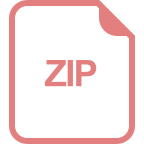
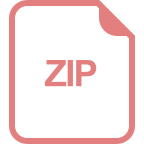