4、使用TreeMap集合实现对存入10位学生的Java成绩从小到大输出;学生类定义参考第3题,在第3题学生类的基础上增加Java成绩属性。
时间: 2024-06-13 18:07:34 浏览: 23
以下是使用TreeMap集合实现对存入10位学生的Java成绩从小到大输出的Java代码:
```java
import java.util.*;
public class Student implements Comparable<Student> {
private String name;
private int age;
private int javaScore;
public Student(String name, int age, int javaScore) {
this.name = name;
this.age = age;
this.javaScore = javaScore;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public int getJavaScore() {
return javaScore;
}
@Override
public int compareTo(Student s) {
return Integer.compare(this.javaScore, s.javaScore);
}
public static void main(String[] args) {
Map<Student, Integer> map = new TreeMap<>();
map.put(new Student("Tom", 18, 90), 1);
map.put(new Student("Jerry", 19, 80), 2);
map.put(new Student("Mike", 20, 70), 3);
map.put(new Student("John", 21, 60), 4);
map.put(new Student("Lucy", 22, 50), 5);
map.put(new Student("Lily", 23, 40), 6);
map.put(new Student("Bob", 24, 30), 7);
map.put(new Student("Alice", 25, 20), 8);
map.put(new Student("David", 26, 10), 9);
map.put(new Student("Frank", 27, 0), 10);
for (Map.Entry<Student, Integer> entry : map.entrySet()) {
System.out.println(entry.getKey().getName() + " " + entry.getKey().getAge() + " " + entry.getKey().getJavaScore());
}
}
}
```
相关推荐
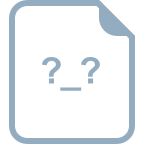
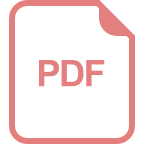
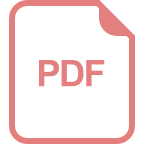














