通过匿名管道实现父子进程间的通信,实现如下功能: 父进程给子进程发信息:fighting,child,you are my hope! 子进程给父进程发信息:fighting,parent,you are my example!
时间: 2024-03-01 20:52:08 浏览: 30
好的,这个问题其实可以通过在父进程中创建匿名管道,并在fork后将管道文件描述符传递给子进程来实现。
以下是一个简单的实现示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
int main()
{
int pipefd[2];
pid_t pid;
char buf[100];
if (pipe(pipefd) == -1) {
perror("pipe");
exit(EXIT_FAILURE);
}
pid = fork();
if (pid == -1) {
perror("fork");
exit(EXIT_FAILURE);
}
if (pid == 0) { /* 子进程 */
close(pipefd[1]); /* 关闭写端 */
read(pipefd[0], buf, sizeof(buf));
printf("%s\n", buf);
sprintf(buf, "fighting, parent, you are my example!");
write(pipefd[1], buf, strlen(buf) + 1);
close(pipefd[0]);
exit(EXIT_SUCCESS);
} else { /* 父进程 */
close(pipefd[0]); /* 关闭读端 */
sprintf(buf, "fighting, child, you are my hope!");
write(pipefd[1], buf, strlen(buf) + 1);
close(pipefd[1]);
wait(NULL);
read(pipefd[0], buf, sizeof(buf));
printf("%s\n", buf);
exit(EXIT_SUCCESS);
}
}
```
这个程序中首先通过pipe函数创建了一个管道,然后通过fork函数创建了一个子进程。
在子进程中,首先关闭了写端,然后通过read函数读取了父进程发送过来的信息并打印出来。接着,使用sprintf函数构造了一个回复信息,并通过write函数写入管道中。最后,关闭了读端并退出。
在父进程中,首先关闭了读端,然后使用sprintf函数构造了一个信息,并通过write函数写入管道中。接着,关闭了写端,等待子进程退出并回收资源。最后,通过read函数读取了子进程发送过来的信息并打印出来,然后退出。
这样,父子进程之间就可以通过管道实现双向通信了。
相关推荐
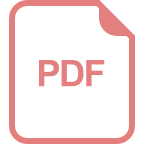
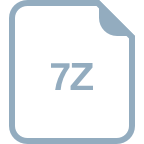
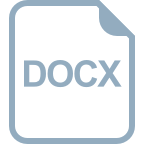














