使用vue编写简易分页器
时间: 2024-01-18 12:10:28 浏览: 76
好的,下面是一个简单的 Vue 分页器组件示例:
```
<template>
<div class="pagination">
<button
v-if="currentPage !== 1"
@click="currentPage--"
>
上一页
</button>
<button
v-for="page in pagesToShow"
:key="page"
:class="{ active: page === currentPage }"
@click="currentPage = page"
>
{{ page }}
</button>
<button
v-if="currentPage !== totalPages"
@click="currentPage++"
>
下一页
</button>
</div>
</template>
<script>
export default {
props: {
totalPages: {
type: Number,
required: true
},
currentPage: {
type: Number,
default: 1
},
pagesToShow: {
type: Number,
default: 5
}
},
computed: {
pages() {
const pagesArray = [];
for (let i = 1; i <= this.totalPages; i++) {
pagesArray.push(i);
}
return pagesArray;
},
pagesInRange() {
const startPage = this.currentPage - Math.floor(this.pagesToShow / 2);
const endPage = this.currentPage + Math.floor(this.pagesToShow / 2);
return this.pages.filter(page => page >= startPage && page <= endPage);
}
}
};
</script>
<style>
.pagination {
display: flex;
justify-content: center;
align-items: center;
}
button {
margin: 0 5px;
padding: 5px 10px;
background-color: #fff;
border: 1px solid #ccc;
border-radius: 3px;
cursor: pointer;
}
button.active {
background-color: #007bff;
color: #fff;
border-color: #007bff;
}
</style>
```
在这个示例中,我们定义了一个名为 `pagination` 的组件,它接受三个 props:`totalPages`,`currentPage` 和 `pagesToShow`。`totalPages` 表示总页数,`currentPage` 表示当前页,`pagesToShow` 表示要显示的页码数量(默认为 5)。
在模板中,我们使用 `v-if` 和 `v-for` 指令来显示上一页、下一页和页码按钮。我们使用 `@click` 监听器来响应按钮的点击事件,并将 `currentPage` 设置为相应的页码。
在计算属性中,我们使用 `pages` 数组来生成所有页码。然后,我们使用 `pagesInRange` 计算属性来生成应该显示的页码范围。我们使用当前页和 `pagesToShow` 计算出范围的起始和结束页码,并使用 `filter` 方法从 `pages` 数组中选择应该显示的页码。
最后,我们定义了一些 CSS 样式来使分页器看起来更漂亮。
阅读全文
相关推荐
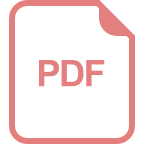
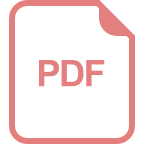

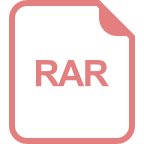
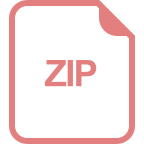
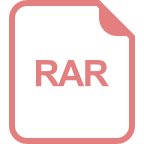
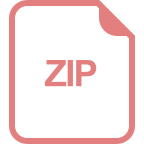
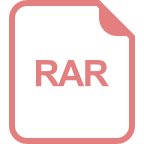
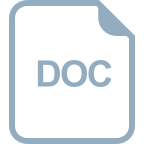
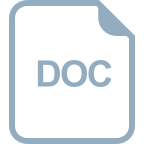
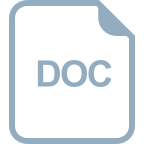
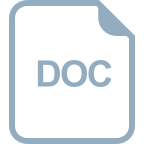
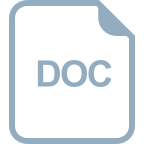
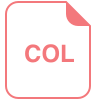