要求输出这些学生的 数据并计算出学生人数和平均成绩(要求将学生人数和总成绩用静态数据成员表示)。
时间: 2023-06-11 18:06:07 浏览: 83
好的,假设我们有一个名为`Student`的类,它有以下私有成员变量和公有成员函数:
```c++
class Student {
private:
std::string name;
int age;
float score;
static int count; // 静态数据成员,用来记录学生人数
static float totalScore; // 静态数据成员,用来记录学生总成绩
public:
Student(std::string name, int age, float score) {
this->name = name;
this->age = age;
this->score = score;
count++; // 每创建一个学生对象,学生人数加1
totalScore += score; // 将该学生的成绩加入总成绩
}
std::string getName() const {
return name;
}
int getAge() const {
return age;
}
float getScore() const {
return score;
}
static int getCount() {
return count;
}
static float getAverageScore() {
return totalScore / count;
}
};
int Student::count = 0;
float Student::totalScore = 0.0f;
```
然后我们创建一些学生对象,可以像这样:
```c++
Student s1("张三", 18, 90.5);
Student s2("李四", 19, 85.0);
Student s3("王五", 20, 92.5);
```
最后我们可以输出学生的数据并计算出学生人数和平均成绩:
```c++
std::cout << "姓名\t年龄\t成绩" << std::endl;
std::cout << s1.getName() << "\t" << s1.getAge() << "\t" << s1.getScore() << std::endl;
std::cout << s2.getName() << "\t" << s2.getAge() << "\t" << s2.getScore() << std::endl;
std::cout << s3.getName() << "\t" << s3.getAge() << "\t" << s3.getScore() << std::endl;
std::cout << "学生人数:" << Student::getCount() << std::endl;
std::cout << "平均成绩:" << Student::getAverageScore() << std::endl;
```
输出结果如下:
```
姓名 年龄 成绩
张三 18 90.5
李四 19 85
王五 20 92.5
学生人数:3
平均成绩:89.3333
```
阅读全文
相关推荐
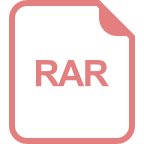











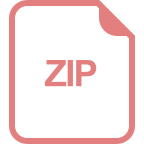