蚁群算法的收获和感想
时间: 2024-04-01 12:27:27 浏览: 96
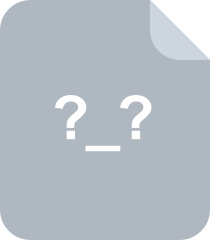
蚁群算法
蚁群算法是一种基于蚂蚁觅食行为的启发式算法,它模拟了蚂蚁在寻找食物时的行为,通过蚂蚁之间的信息交流和合作,最终找到最优解。我学习蚁群算法的过程中,有以下几点收获和感想:
1. 蚁群算法的思想很有启发性,它让我意识到了合作和信息交流的重要性,这对于解决很多实际问题都有很大的借鉴意义。
2. 蚁群算法的实现过程中需要考虑很多因素,如信息素的更新、蚂蚁的移动策略等,这让我更加深入地理解了算法的本质和实现细节。
3. 在实现蚁群算法的过程中,我也学会了如何使用Python进行数据可视化,这让我更加直观地了解算法的运行过程和结果。
以下是一个简单的蚁群算法实现的例子,用于解决旅行商问题:
```python
# 导入必要的库
import numpy as np
import matplotlib.pyplot as plt
# 定义城市坐标
cities = np.array([[60, 200], [180, 200], [80, 180], [140, 180], [20, 160],
[100, 160], [200, 160], [140, 140], [40, 120], [100, 120],
[180, 100], [60, 80], [120, 80], [180, 60], [20, 40],
[100, 40], [200, 40], [20, 20], [60, 20], [160, 20]])
# 计算城市之间的距离
def distance(city1, city2):
return np.sqrt(np.sum((city1 - city2) ** 2))
num_cities = len(cities)
distances = np.zeros((num_cities, num_cities))
for i in range(num_cities):
for j in range(num_cities):
distances[i][j] = distance(cities[i], cities[j])
# 定义蚂蚁类
class Ant:
def __init__(self, alpha, beta, num_cities, distances):
self.alpha = alpha
self.beta = beta
self.num_cities = num_cities
self.distances = distances
self.pheromone = np.ones((num_cities, num_cities)) / num_cities
self.visited = np.zeros(num_cities, dtype=bool)
self.tour = np.zeros(num_cities, dtype=int)
self.tour_length = 0
# 选择下一个城市
def select_next_city(self, current_city):
unvisited_cities = np.where(self.visited == False)[0]
pheromone = np.power(self.pheromone[current_city][unvisited_cities], self.alpha)
distance = np.power(1 / self.distances[current_city][unvisited_cities], self.beta)
prob = pheromone * distance / np.sum(pheromone * distance)
next_city = np.random.choice(unvisited_cities, p=prob)
return next_city
# 蚂蚁移动
def move(self, start_city):
self.visited[start_city] = True
self.tour[0] = start_city
for i in range(1, self.num_cities):
current_city = self.tour[i - 1]
next_city = self.select_next_city(current_city)
self.visited[next_city] = True
self.tour[i] = next_city
self.tour_length += self.distances[current_city][next_city]
self.tour_length += self.distances[self.tour[-1]][self.tour[0]]
# 更新信息素
def update_pheromone(self, pheromone_decay):
delta_pheromone = np.zeros((self.num_cities, self.num_cities))
for i in range(self.num_cities):
j = (i + 1) % self.num_cities
delta_pheromone[self.tour[i]][self.tour[j]] += 1 / self.tour_length
self.pheromone = (1 - pheromone_decay) * self.pheromone + pheromone_decay * delta_pheromone
# 定义蚁群算法类
class AntColony:
def __init__(self, num_ants, alpha, beta, pheromone_decay, num_iterations, num_cities, distances):
self.num_ants = num_ants
self.alpha = alpha
self.beta = beta
self.pheromone_decay = pheromone_decay
self.num_iterations = num_iterations
self.num_cities = num_cities
self.distances = distances
self.best_tour = np.zeros(num_cities, dtype=int)
self.best_tour_length = np.inf
# 运行蚁群算法
def run(self):
for iteration in range(self.num_iterations):
ants = [Ant(self.alpha, self.beta, self.num_cities, self.distances) for i in range(self.num_ants)]
for ant in ants:
ant.move(np.random.randint(self.num_cities))
if ant.tour_length < self.best_tour_length:
self.best_tour_length = ant.tour_length
self.best_tour = ant.tour
ant.update_pheromone(self.pheromone_decay)
# 绘制最优路径
plt.figure()
plt.scatter(cities[:, 0], cities[:, 1])
for i in range(num_cities - 1):
plt.plot([cities[self.best_tour[i], 0], cities[self.best_tour[i + 1], 0]],
[cities[self.best_tour[i], 1], cities[self.best_tour[i + 1], 1]], 'r')
plt.plot([cities[self.best_tour[0], 0], cities[self.best_tour[-1], 0]],
[cities[self.best_tour[0], 1], cities[self.best_tour[-1], 1]], 'r')
plt.show()
# 运行蚁群算法
num_ants = 50
alpha = 1
beta = 5
pheromone_decay = 0.5
num_iterations = 100
ant_colony = AntColony(num_ants, alpha, beta, pheromone_decay, num_iterations, num_cities, distances)
ant_colony.run()
```
阅读全文
相关推荐
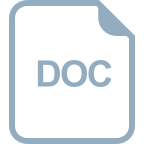
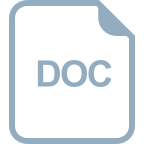
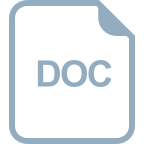
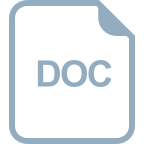
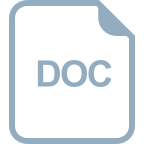
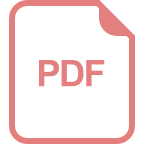
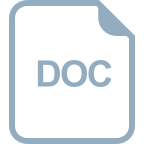
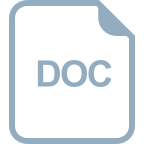
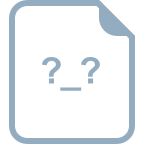
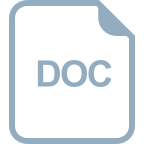
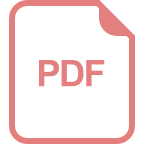