stm32不用定时器轮询的方式实现长按和短按并把代码写出来加注释
时间: 2024-09-29 21:12:06 浏览: 28
在STM32中,如果不想使用定时器进行轮询,可以通过硬件触发的中断来实现按键的长按和短按。这里提供一个基础的示例,假设我们有一个按键Pin K1,我们将使用 EXTI 中断来检测按键的按下和释放状态:
```c
#include "stm32f10x.h" // Include your STM32 HAL header
// Define the GPIO and EXTI configuration for the key
GPIO_InitTypeDef GPIO_InitStructure;
EXTI_HandleTypeDef EXTI_InitStructure;
// Key pin configuration (replace 'GPIOA_Pin_1' with your actual pin)
#define KEY_PIN GPIO_PIN_1
#define KEY_PORT GPIOA
void EXTI_K1_IRQHandler(void) {
// EXTI line detection
if(__HAL_GPIO_EXTI_GET_IT(KEY_PORT, KEY_PIN)) {
__HAL_GPIO_EXTI_CLEAR_IT(KEY_PORT, KEY_PIN); // Clear interrupt flag
// Check key state - if it's pressed
if(__HAL_GPIO_ReadPin(KEY_PORT, KEY_PIN)) {
// Long press check using a flag or a variable (e.g., a counter)
static uint8_t longPressCount = 0; // Counter to count presses
++longPressCount;
if(longPressCount > MIN_PRESS_TIMES) { // If it's been pressed enough times
// Handle long press here
// ...
longPressCount = 0; // Reset for next potential long press
} else {
// Short press handling
// ...
}
} else {
// Release detected, reset counter if needed
longPressCount = 0;
}
}
}
int main(void) {
// Initialize GPIO and EXTI
GPIO_InitStructure.Pin = KEY_PIN;
GPIO_InitStructure.Mode = GPIO_MODE_INPUT;
GPIO_InitStructure.Pull = GPIO_NOPULL;
HAL_GPIO_Init(KEY_PORT, &GPIO_InitStructure);
EXTI_InitStructure.Line = KEY_PIN;
EXTI_InitStructure.Type = EXTI_TYPE Rising; // Detect rising edge (key pressing)
EXTI_InitStructure.Polarity = EXTI_POLARITY_RISING;
EXTI_InitStructure.Interrupt = EXTI_INTERRUPT_ENABLE;
HAL_GPIO_EXTI_Init(&EXTI_InitStructure);
// Enable global interrupt
HAL_NVIC_EnableIRQ(EXTI0_IRQn); // EXTI0_IRQn corresponds to GPIOA
while (1) {
// Your other main loop tasks
}
return 0;
}
```
相关推荐
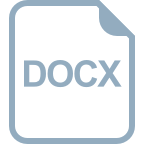
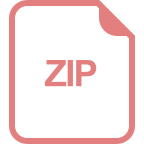
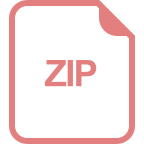
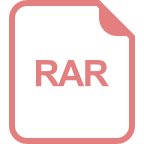
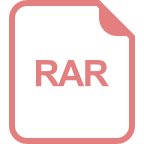
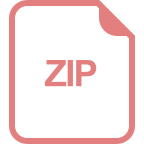
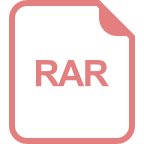
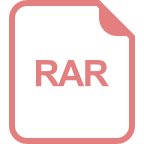
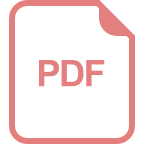
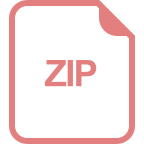
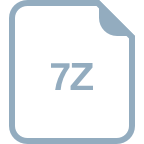
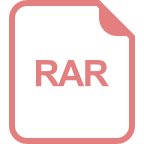
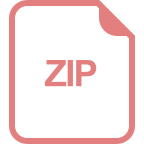
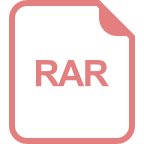
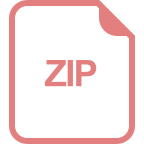
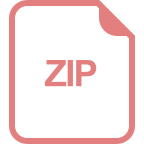
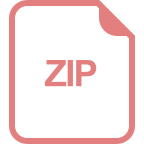
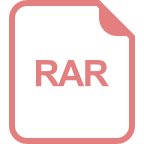