js中如何判断字符串中是否包含https
时间: 2023-10-27 15:04:39 浏览: 24
可以使用JavaScript中的`includes()`或`indexOf()`方法来判断一个字符串中是否包含另一个字符串。
例如,可以使用以下代码来判断一个字符串`str`中是否包含`https`:
```javascript
if (str.includes('https')) {
console.log('字符串中包含https');
}
// 或者
if (str.indexOf('https') !== -1) {
console.log('字符串中包含https');
}
```
这两种方法都会返回一个布尔值,如果字符串中包含指定的子字符串,则返回`true`,否则返回`false`。
相关问题
JS中判断字符串A是否包含字符串B
要判断字符串A是否包含字符串B,可以使用JavaScript中的`includes()`方法或者正则表达式。
1. 使用`includes()`方法:
```javascript
const A = "Hello World";
const B = "World";
if (A.includes(B)) {
console.log("A包含B");
} else {
console.log("A不包含B");
}
```
2. 使用正则表达式:
```javascript
const A = "Hello World";
const B = "World";
const regex = new RegExp(B);
if (regex.test(A)) {
console.log("A包含B");
} else {
console.log("A不包含B");
}
```
这两种方法都可以用来判断字符串A是否包含字符串B。注意,`includes()`方法在ES6中引入,而正则表达式可以提供更多的匹配灵活性。
js 正则判断字符串中是否包含某个字符串
在JavaScript中,可以使用正则表达式的`test()`方法来判断一个字符串是否包含某个特定的字符或字符串。`test()`方法会返回一个布尔值,如果字符串中包含了指定的字符或字符串,则返回true,否则返回false。下面是一个示例代码:
```javascript
var str = "Hello World";
var pattern = /World/;
if (pattern.test(str)) {
console.log("字符串包含指定的字符或字符串");
} else {
console.log("字符串不包含指定的字符或字符串");
}
```
在上面的例子中,我们使用正则表达式`/World/`来判断字符串`str`是否包含了`World`这个字符串。如果包含,就会输出`字符串包含指定的字符或字符串`,否则输出`字符串不包含指定的字符或字符串`。
相关推荐
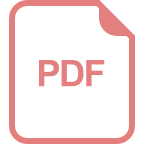
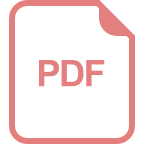
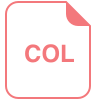
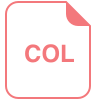
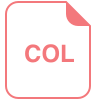
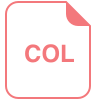
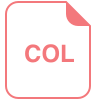







