spring boot通过ftp获取pdf文件存储到内存然后直接给前端下载
时间: 2024-10-25 20:10:39 浏览: 13
Spring Boot 可以通过 FTP 服务连接远程服务器,获取 PDF 文件,并将其内容读取到内存中。这通常涉及到几个步骤:
1. **添加依赖**:首先,在你的 Spring Boot 项目中添加 FTP 连接库,如 Apache Commons Net 或者 Spring Framework 自带的 `spring-boot-starter-netty`。
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-netty</artifactId>
</dependency>
```
2. **配置 FTP 连接**:创建一个 FTP 配置类,设置 FTP 服务器的地址、用户名、密码等信息。
```java
@Configuration
public class FtpConfig {
@Value("${ftp.host}")
private String host;
@Value("${ftp.port}")
private int port;
@Value("${ftp.username}")
private String username;
@Value("${ftp.password}")
private String password;
@Bean
public SimpleFtpClient ftpClient() throws Exception {
SimpleFtpClient client = new SimpleFtpClient();
client.setHost(host);
client.setPort(port);
client.login(username, password);
return client;
}
}
```
3. **FTP 文件操作**:编写一个方法来从 FTP 获取 PDF 文件,例如使用 `SimpleFtpClient` 的 `retrieveFile()` 方法。
```java
@Service
public class PdfDownloaderService {
private final FtpClient ftpClient;
public PdfDownloaderService(FtpConfig ftpConfig) {
this.ftpClient = ftpConfig.ftpClient();
}
@GetMapping("/download-pdf")
public byte[] downloadPdfFromFtp(String fileName) throws IOException {
InputStream inputStream = ftpClient.retrieveFileStream(fileName);
try (BufferedInputStream bis = new BufferedInputStream(inputStream)) {
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = bis.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
return outputStream.toByteArray();
} finally {
inputStream.close();
}
}
}
```
4. **前端处理**:在前端,你可以将返回的字节数组转换为 Blob 对象,然后提供下载链接。
5. **错误处理**:别忘了处理可能出现的异常,比如网络错误、权限问题等。
**相关问题--:**
1. 如何处理FTP连接失败的情况?
2. 怎么避免频繁访问FTP服务器导致的性能问题?
3. 使用Spring Boot的Netty版本是否比普通的FTP客户端更高效?
阅读全文
相关推荐
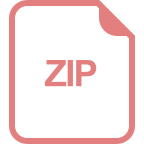
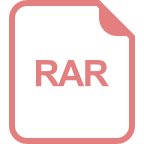
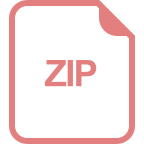



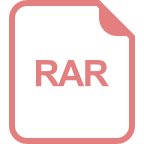
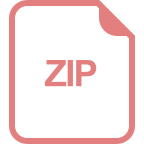
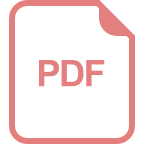
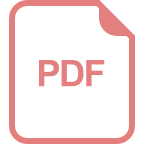
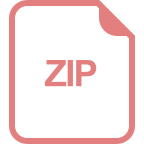
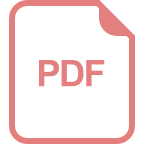
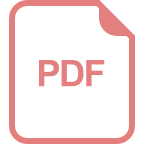
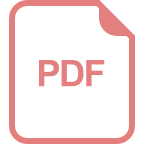
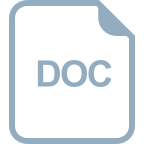
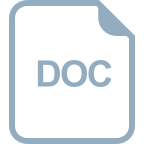
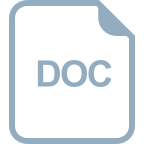


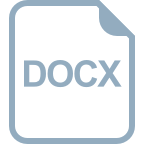