被Maven识别的jar包:thymeleaf-extras-java8time
时间: 2024-10-18 20:20:42 浏览: 35
Thymeleaf-extras-java8time是一个Thymeleaf模板引擎的扩展模块,它允许在Thymeleaf模板中直接使用Java 8中的日期和时间API。Maven是一个构建工具,通过它你可以轻松地管理项目依赖,包括添加、删除和升级第三方库。当你在Maven的pom.xml文件中声明`thymeleaf-extras-java8time`作为项目的依赖时,Maven会自动下载并存放在本地仓库中,供构建过程使用。
例如,在pom.xml中可能会有类似这样的配置:
```xml
<dependency>
<groupId>org.thymeleaf.extras</groupId>
<artifactId>thymeleaf-extras-java8time</artifactId>
<version>3.0.13.RELEASE</version>
</dependency>
```
这里的`groupId`是组织ID,`artifactId`是项目ID,`version`是版本号。当你运行`mvn install`或`mvn compile`等命令时,Maven就会处理这个依赖。
相关问题
代码实现一个基于Spring boot + Mybatis-Plus+Thymeleaf+Bootstrap 的留言板
好的,下面我将为你展示如何实现一个基于Spring Boot、Mybatis-Plus、Thymeleaf和Bootstrap的留言板。
1. 创建Spring Boot项目
首先,我们需要创建一个Spring Boot项目。你可以直接在IDE中创建,也可以使用Spring Boot CLI。这里我使用IDEA创建一个Maven项目。
2. 配置pom.xml
在pom.xml文件中添加以下依赖:
```
<dependencies>
<!-- Spring Boot Starter -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Thymeleaf -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<!-- MyBatis-Plus -->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.1.1</version>
</dependency>
<!-- MySQL Connector -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.21</version>
</dependency>
<!-- Bootstrap -->
<dependency>
<groupId>org.webjars</groupId>
<artifactId>bootstrap</artifactId>
<version>4.5.0</version>
</dependency>
<!-- Webjars Locator -->
<dependency>
<groupId>org.webjars</groupId>
<artifactId>webjars-locator</artifactId>
<version>0.40</version>
</dependency>
</dependencies>
```
这里使用了Spring Boot、Thymeleaf、Mybatis-Plus、MySQL Connector、Bootstrap以及Webjars Locator。
3. 配置数据库
我们需要在application.properties文件中配置数据库连接信息。
```
spring.datasource.url=jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=UTF-8&useSSL=false&serverTimezone=UTC
spring.datasource.username=root
spring.datasource.password=root
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
```
这里使用MySQL作为数据库,数据库名为test,用户名和密码均为root。
4. 创建留言实体类
我们需要创建一个留言实体类,用于映射数据库中的留言表。
```
public class Message {
private Long id;
private String username;
private String content;
private Date createTime;
// 省略getter和setter方法
}
```
5. 创建Mapper
我们需要创建一个Mapper接口,用于操作留言表。
```
@Mapper
public interface MessageMapper extends BaseMapper<Message> {
List<Message> selectAll();
}
```
这里使用了Mybatis-Plus提供的BaseMapper作为父接口,简化了Mapper的编写。
6. 创建Controller
我们需要创建一个Controller,用于处理请求。
```
@Controller
public class MessageController {
@Autowired
private MessageMapper messageMapper;
@GetMapping("/")
public String index(Model model) {
List<Message> messages = messageMapper.selectAll();
model.addAttribute("messages", messages);
return "index";
}
@PostMapping("/")
public String addMessage(Message message) {
message.setCreateTime(new Date());
messageMapper.insert(message);
return "redirect:/";
}
}
```
这里使用了@Autowired注解注入了MessageMapper。在index方法中,我们查询了所有留言,并将其存入Model中,然后返回index视图。在addMessage方法中,我们添加了一条留言,并重定向到首页。
7. 创建视图
我们需要创建一个视图,用于显示留言列表和添加留言。
```
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org"
xmlns:webjars="http://www.thymeleaf.org/extras/webjar">
<head>
<title>留言板</title>
<!-- Bootstrap -->
<link rel="stylesheet" href="/webjars/bootstrap/4.5.0/css/bootstrap.min.css" />
</head>
<body>
<div class="container">
<h1>留言板</h1>
<hr/>
<form method="post">
<div class="form-group">
<label for="username">用户名:</label>
<input type="text" class="form-control" id="username" name="username" required="required" />
</div>
<div class="form-group">
<label for="content">留言内容:</label>
<textarea class="form-control" id="content" name="content" required="required"></textarea>
</div>
<button type="submit" class="btn btn-primary">提交留言</button>
</form>
<hr/>
<table class="table table-striped">
<thead>
<tr>
<th>用户名</th>
<th>留言内容</th>
<th>留言时间</th>
</tr>
</thead>
<tbody>
<tr th:each="message : ${messages}">
<td th:text="${message.username}"></td>
<td th:text="${message.content}"></td>
<td th:text="${#dates.format(message.createTime, 'yyyy-MM-dd HH:mm:ss')}"></td>
</tr>
</tbody>
</table>
</div>
<!-- Bootstrap -->
<script src="/webjars/bootstrap/4.5.0/js/bootstrap.min.js"></script>
</body>
</html>
```
这里使用了Thymeleaf模板引擎,使用了Bootstrap样式。在表格中,我们使用了th:each指令循环遍历留言列表,使用了#dates.format方法格式化留言时间。
8. 运行项目
现在,我们可以运行项目并访问http://localhost:8080来查看留言板了。
以上就是一个简单的Spring Boot、Mybatis-Plus、Thymeleaf和Bootstrap留言板的实现过程。希望对你有帮助。
阅读全文
相关推荐
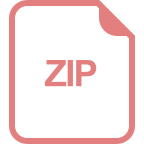
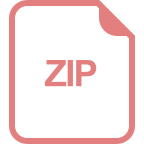
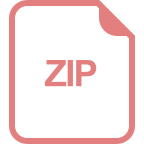
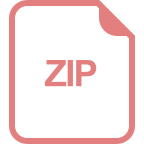
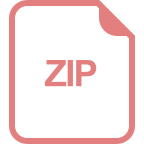
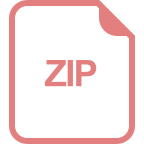
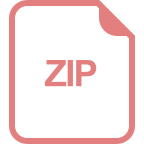
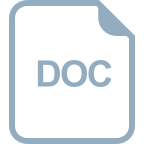
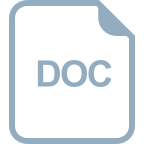
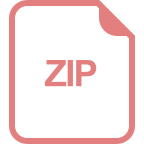
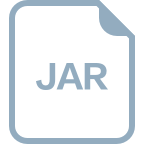
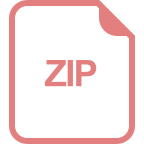
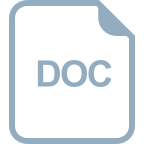
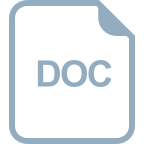
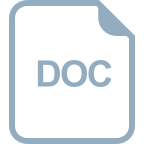
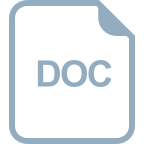
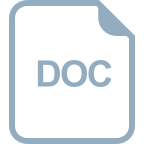
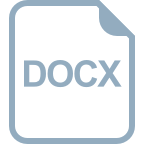