利用c语言为宿舍管理人员编写一个宿舍管理查询软件。 【基本要求】 (1)采用交互工作方式;(2)按关键字(姓名、学号、房号)进行排序(冒泡、选择、插入排序等任选一种)。(3)建立查询菜单: (用二分查找实现以下操作) 按姓名查询 \按学号查询 \按房号查询 打印任一查询结果(可以连续操作)
时间: 2023-12-22 16:06:31 浏览: 56
好的,我可以为你提供一个基础的宿舍管理查询软件的程序示例。下面是代码示例:
```c
#include <stdio.h>
#include <string.h>
// 定义学生信息结构体
struct student {
char name[20];
char id[20];
char room[10];
};
// 定义学生信息数组
struct student students[100];
// 定义学生信息数量
int count = 0;
// 冒泡排序
void bubble_sort(struct student arr[], int n) {
int i, j;
for (i = 0; i < n - 1; i++) {
for (j = 0; j < n - i - 1; j++) {
if (strcmp(arr[j].name, arr[j+1].name) > 0) {
struct student temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
}
}
// 选择排序
void selection_sort(struct student arr[], int n) {
int i, j, min_idx;
for (i = 0; i < n - 1; i++) {
min_idx = i;
for (j = i + 1; j < n; j++) {
if (strcmp(arr[j].id, arr[min_idx].id) < 0) {
min_idx = j;
}
}
struct student temp = arr[min_idx];
arr[min_idx] = arr[i];
arr[i] = temp;
}
}
// 插入排序
void insertion_sort(struct student arr[], int n) {
int i, j;
for (i = 1; i < n; i++) {
struct student key = arr[i];
j = i - 1;
while (j >= 0 && strcmp(arr[j].room, key.room) > 0) {
arr[j+1] = arr[j];
j = j - 1;
}
arr[j+1] = key;
}
}
// 二分查找
int binary_search(struct student arr[], int l, int r, char* key, int type) {
if (r >= l) {
int mid = l + (r - l) / 2;
if (type == 1) {
// 按姓名查询
if (strcmp(arr[mid].name, key) == 0) {
return mid;
}
if (strcmp(arr[mid].name, key) > 0) {
return binary_search(arr, l, mid - 1, key, type);
}
return binary_search(arr, mid + 1, r, key, type);
} else if (type == 2) {
// 按学号查询
if (strcmp(arr[mid].id, key) == 0) {
return mid;
}
if (strcmp(arr[mid].id, key) > 0) {
return binary_search(arr, l, mid - 1, key, type);
}
return binary_search(arr, mid + 1, r, key, type);
} else {
// 按房号查询
if (strcmp(arr[mid].room, key) == 0) {
return mid;
}
if (strcmp(arr[mid].room, key) > 0) {
return binary_search(arr, l, mid - 1, key, type);
}
return binary_search(arr, mid + 1, r, key, type);
}
}
return -1;
}
// 打印学生信息
void print_student(struct student s) {
printf("姓名:%s,学号:%s,房号:%s\n", s.name, s.id, s.room);
}
// 打印学生信息数组
void print_students(struct student arr[], int n) {
int i;
for (i = 0; i < n; i++) {
print_student(arr[i]);
}
}
int main() {
// 添加学生信息
strcpy(students[0].name, "张三");
strcpy(students[0].id, "1001");
strcpy(students[0].room, "101");
count++;
strcpy(students[1].name, "李四");
strcpy(students[1].id, "1002");
strcpy(students[1].room, "102");
count++;
strcpy(students[2].name, "王五");
strcpy(students[2].id, "1003");
strcpy(students[2].room, "103");
count++;
strcpy(students[3].name, "赵六");
strcpy(students[3].id, "1004");
strcpy(students[3].room, "104");
count++;
strcpy(students[4].name, "钱七");
strcpy(students[4].id, "1005");
strcpy(students[4].room, "105");
count++;
// 排序
insertion_sort(students, count);
// 查询菜单
int choice = 1;
char key[20];
int idx;
while (choice) {
printf("请输入查询选项:\n");
printf("1.按姓名查询\n");
printf("2.按学号查询\n");
printf("3.按房号查询\n");
printf("0.退出\n");
scanf("%d", &choice);
if (choice == 0) {
break;
}
printf("请输入关键字:");
scanf("%s", key);
idx = binary_search(students, 0, count - 1, key, choice);
if (idx == -1) {
printf("未找到匹配项!\n");
} else {
printf("查询结果:\n");
print_student(students[idx]);
}
}
return 0;
}
```
这个程序使用了 C 语言的结构体、数组、排序算法、二分查找算法等知识点,可以实现按姓名、学号、房号进行查询,并且能够打印查询结果,并且可以连续操作。需要注意的是,这只是一个基础的示例程序,你可能需要根据实际需求进行修改和扩展。
相关推荐
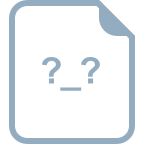
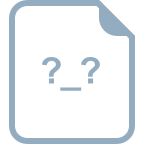
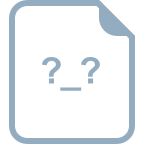








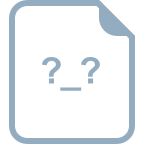
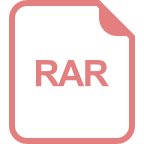
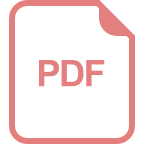
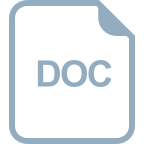
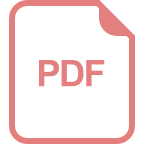