js 实现 自定义的弹出式确认窗口的代码
时间: 2024-03-05 12:53:38 浏览: 70
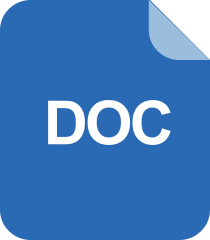
弹出窗口的创建
实现自定义的弹出式确认窗口需要用到 HTML、CSS 和 JavaScript。以下是一个简单的实现例子:
HTML 代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>Custom Confirm Box</title>
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<h1>Custom Confirm Box Example</h1>
<button onclick="showConfirm()">Show Confirm Box</button>
<div id="confirm-box">
<div id="confirm-message"></div>
<div id="confirm-buttons">
<button id="confirm-yes" onclick="confirmYes()">Yes</button>
<button id="confirm-no" onclick="confirmNo()">No</button>
</div>
</div>
<script src="script.js"></script>
</body>
</html>
```
CSS 代码:
```css
#confirm-box {
display: none;
position: fixed;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
background-color: #fff;
border: 1px solid #ccc;
padding: 20px;
}
#confirm-message {
margin-bottom: 20px;
}
#confirm-buttons {
text-align: right;
}
#confirm-yes, #confirm-no {
margin-left: 10px;
}
```
JavaScript 代码:
```js
var confirmBox = document.getElementById("confirm-box");
var confirmMessage = document.getElementById("confirm-message");
var confirmYes = document.getElementById("confirm-yes");
var confirmNo = document.getElementById("confirm-no");
function showConfirm() {
confirmBox.style.display = "block";
confirmMessage.innerHTML = "Are you sure you want to delete this item?";
}
function confirmYes() {
confirmBox.style.display = "none";
alert("Item deleted successfully!");
}
function confirmNo() {
confirmBox.style.display = "none";
alert("Delete operation cancelled.");
}
```
在这个例子中,我们创建了一个自定义的确认框。当用户点击 "Show Confirm Box" 按钮时,会弹出一个确认框,询问用户是否确定删除某个项目。如果用户点击确认按钮,则会显示一个警告框,表示项目已经成功删除。如果用户点击取消按钮,则会显示一个警告框,表示删除操作已被取消。我们使用 CSS 对确认框进行样式设置,使用 JavaScript 来控制确认框的显示和隐藏,以及处理确认和取消操作。
阅读全文
相关推荐
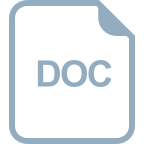
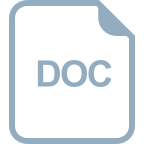

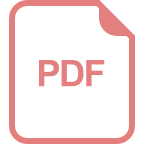
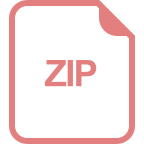
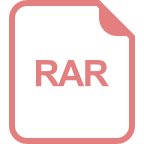
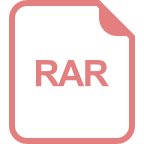
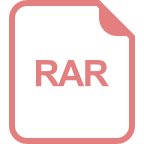
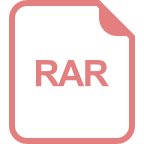
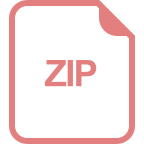
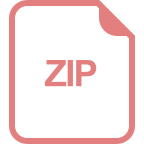
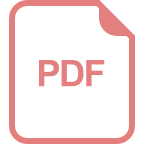
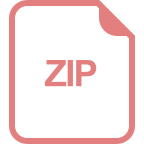
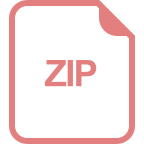
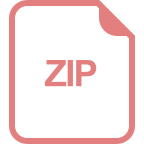
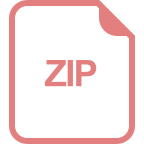
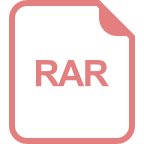
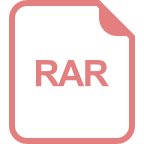