vue3 tinymce获取到的html文件上传到后端node服务器 报错413请求体过大怎么解决
时间: 2024-03-09 14:49:18 浏览: 28
413请求体过大的错误提示通常是由于上传的数据量大于服务器所允许的最大值,导致服务器拒绝接受请求。
为了解决这个问题,你可以尝试以下几种方法:
1. 修改服务器的上传文件大小限制
在 Node.js 中,可以通过增加 `body-parser` 模块的 `limit` 属性来修改上传文件大小的限制。例如,将上传文件大小限制改为 100MB:
```javascript
const bodyParser = require('body-parser');
app.use(bodyParser.json({limit: '100mb', extended: true}));
app.use(bodyParser.urlencoded({limit: '100mb', extended: true}));
```
2. 将上传数据进行分块上传
将上传的数据进行分块上传,每次上传一部分数据,直到全部上传完成。可以使用 `Blob` 对象来进行分块上传,前端代码示例如下:
```javascript
const chunkSize = 1024 * 1024; // 分块大小为 1MB
const totalChunks = Math.ceil(file.size / chunkSize); // 总共需要上传的分块数
let currentChunk = 0; // 当前上传的分块编号
while (currentChunk < totalChunks) {
const start = currentChunk * chunkSize;
const end = Math.min(start + chunkSize, file.size);
const chunk = file.slice(start, end); // 分割文件
// 将分块上传到服务器
const formData = new FormData();
formData.append('file', chunk, `${file.name}.${currentChunk}`);
formData.append('totalChunks', totalChunks);
formData.append('currentChunk', currentChunk);
// 发送上传请求
await axios.post('/upload', formData);
currentChunk++;
}
```
在服务器端,可以使用 `multiparty` 模块来接收分块上传的数据,合成完整的文件。示例代码:
```javascript
const multiparty = require('multiparty');
app.post('/upload', (req, res) => {
const form = new multiparty.Form();
form.parse(req, (err, fields, files) => {
const currentChunk = fields.currentChunk[0];
const totalChunks = fields.totalChunks[0];
const filename = files.file[0].originalFilename;
const file = files.file[0].path;
// 将分块保存到本地
fs.renameSync(file, `${filename}.${currentChunk}`);
// 如果所有分块都已上传,则合并文件
if (currentChunk == totalChunks - 1) {
const chunks = [];
for (let i = 0; i < totalChunks; i++) {
chunks.push(fs.readFileSync(`${filename}.${i}`));
}
fs.writeFileSync(filename, Buffer.concat(chunks));
res.send('上传完成!');
} else {
res.send(`第 ${currentChunk + 1} 分块上传成功!`);
}
});
});
```
以上是两种解决413请求体过大问题的方法,你可以根据自己的需要选择其中一种。
相关推荐
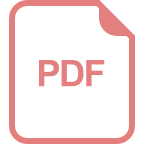














