java评论功能实现代码
时间: 2024-01-02 09:00:26 浏览: 190
以下是一个简单的Java评论功能实现代码,使用了Servlet和JSP技术:
1. 创建一个Comment类,包含评论的属性,如评论内容、评论时间、评论者等。
public class Comment {
private String content;
private String time;
private String author;
public Comment(String content, String time, String author) {
this.content = content;
this.time = time;
this.author = author;
}
// getters and setters
}
2. 创建一个CommentDAO类,用于操作评论数据,包括添加评论、删除评论、获取评论列表等。
public class CommentDAO {
private List<Comment> comments = new ArrayList<>();
public void addComment(Comment comment) {
comments.add(comment);
}
public void deleteComment(int index) {
comments.remove(index);
}
public List<Comment> getComments() {
return comments;
}
}
3. 创建一个Servlet处理评论请求,包括添加评论、删除评论等。
@WebServlet("/comment")
public class CommentServlet extends HttpServlet {
private CommentDAO commentDAO = new CommentDAO();
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String action = request.getParameter("action");
if ("add".equals(action)) {
String content = request.getParameter("content");
String author = request.getParameter("author");
String time = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss").format(new Date());
Comment comment = new Comment(content, time, author);
commentDAO.addComment(comment);
} else if ("delete".equals(action)) {
int index = Integer.parseInt(request.getParameter("index"));
commentDAO.deleteComment(index);
}
response.sendRedirect(request.getContextPath() + "/comment.jsp");
}
}
4. 创建一个JSP页面用于显示评论列表和添加评论。
<%@ page contentType="text/html; charset=UTF-8" %>
<!DOCTYPE html>
<html>
<head>
<title>评论功能</title>
</head>
<body>
<h1>评论列表</h1>
<ul>
<c:forEach items="${commentDAO.comments}" var="comment" varStatus="status">
<li>
${comment.content} - ${comment.author} (${comment.time})
<form method="POST" action="${pageContext.request.contextPath}/comment">
<input type="hidden" name="action" value="delete">
<input type="hidden" name="index" value="${status.index}">
<button>删除</button>
</form>
</li>
</c:forEach>
</ul>
<h1>添加评论</h1>
<form method="POST" action="${pageContext.request.contextPath}/comment">
<input type="hidden" name="action" value="add">
<div>
<label>内容:</label>
<textarea name="content"></textarea>
</div>
<div>
<label>作者:</label>
<input type="text" name="author">
</div>
<button>提交</button>
</form>
</body>
</html>
以上代码只是一个简单的示例,实际的评论功能可能需要更复杂的实现,比如用户登录、评论审核、分页显示等。
阅读全文
相关推荐
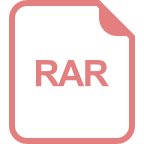
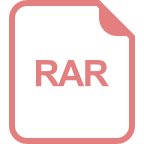
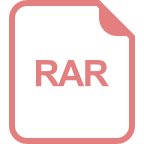
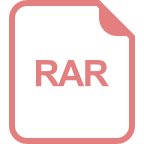
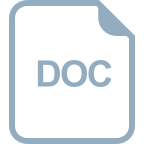
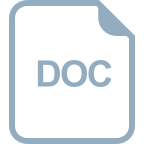
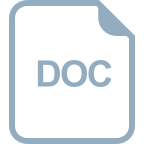