用C++写一个八叉数算法
时间: 2023-05-13 22:05:31 浏览: 379
八叉树算法是一种用于空间划分的数据结构,可以用于三维场景的碰撞检测、光照计算等。以下是一个简单的八叉树算法的实现:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_DEPTH 10
typedef struct {
float x, y, z;
} Point;
typedef struct {
Point min, max;
} AABB;
typedef struct OctreeNode {
AABB bounds;
struct OctreeNode* children[8];
} OctreeNode;
OctreeNode* createOctreeNode(AABB bounds, int depth) {
OctreeNode* node = (OctreeNode*)malloc(sizeof(OctreeNode));
node->bounds = bounds;
for (int i = 0; i < 8; i++) {
node->children[i] = NULL;
}
if (depth < MAX_DEPTH) {
Point center = { (bounds.min.x + bounds.max.x) / 2,
(bounds.min.y + bounds.max.y) / 2,
(bounds.min.z + bounds.max.z) / 2 };
float halfSize = (bounds.max.x - bounds.min.x) / 2;
for (int i = 0; i < 8; i++) {
AABB childBounds = {
.min = { center.x + halfSize * ((i & 1) ? 1 : -1),
center.y + halfSize * ((i & 2) ? 1 : -1),
center.z + halfSize * ((i & 4) ? 1 : -1) },
.max = { center.x + halfSize * ((i & 1) ? -1 : 1),
center.y + halfSize * ((i & 2) ? -1 : 1),
center.z + halfSize * ((i & 4) ? -1 : 1) }
};
node->children[i] = createOctreeNode(childBounds, depth + 1);
}
}
return node;
}
void destroyOctreeNode(OctreeNode* node) {
if (node) {
for (int i = 0; i < 8; i++) {
destroyOctreeNode(node->children[i]);
}
free(node);
}
}
int main() {
AABB bounds = { .min = { -1, -1, -1 }, .max = { 1, 1, 1 } };
OctreeNode* root = createOctreeNode(bounds, 0);
destroyOctreeNode(root);
return 0;
}
```
这个实现中,我们定义了一个 AABB 结构体表示一个三维空间中的轴对齐包围盒,一个 Point 结构体表示一个三维空间中的点。OctreeNode 结构体表示八叉树的一个节点,包含了一个 AABB 表示节点的包围盒,以及一个指向八个子节点的指针数组。createOctreeNode 函数用于创建一个八叉树节点,它接受一个 AABB 表示节点的包围盒,以及一个深度参数。如果深度小于最大深度,它会递归地创建八个子节点,并将它们存储在 children 数组中。destroyOctreeNode 函数用于销毁一个八叉树节点及其所有子节点。在 main 函数中,我们创建了一个根节点,然后销毁它。
阅读全文
相关推荐
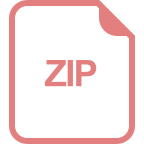
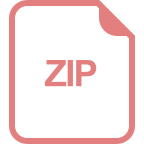
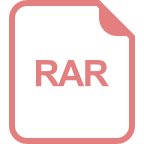
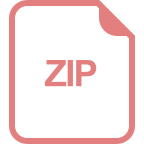
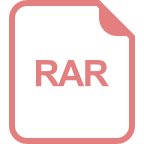
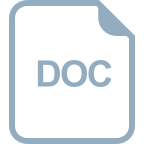

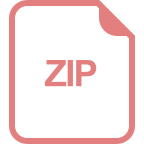
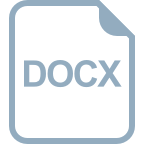
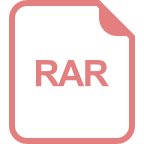
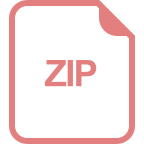
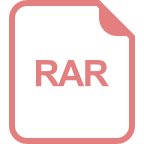
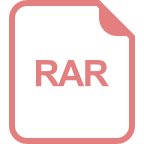
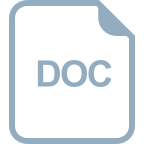
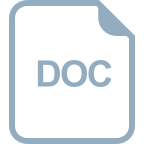