使用dlib人脸关键点检测实现摄像头嘴巴内部轮廓彩色图片的代码,轮廓外透明
时间: 2024-02-23 17:01:51 浏览: 77
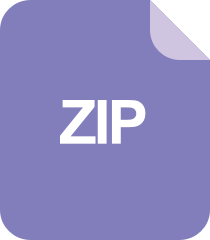
人脸检测功能使用dlib库实现
以下是使用dlib人脸关键点检测实现摄像头嘴巴内部轮廓彩色图片的Python代码:
```python
import cv2
import numpy as np
import dlib
# 加载dlib的人脸检测器和关键点检测器
detector = dlib.get_frontal_face_detector()
predictor = dlib.shape_predictor("shape_predictor_68_face_landmarks.dat")
# 打开摄像头
cap = cv2.VideoCapture(0)
while True:
# 读取摄像头的帧
ret, frame = cap.read()
if not ret:
break
# 转换为灰度图像
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 人脸检测
faces = detector(gray)
# 遍历每个人脸
for face in faces:
# 关键点检测
landmarks = predictor(gray, face)
# 取出嘴巴内部的关键点
mouth_points = []
for i in range(48, 68):
x = landmarks.part(i).x
y = landmarks.part(i).y
mouth_points.append((x, y))
# 创建一个黑色的图像,大小与原始图像相同
mask = np.zeros_like(frame)
# 在黑色图像上绘制填充了白色的嘴巴内部轮廓
cv2.fillPoly(mask, [np.array(mouth_points)], (255, 255, 255))
# 将黑色图像转换为透明图像
alpha = np.zeros_like(mask)
alpha[:, :, 3] = 255 - cv2.cvtColor(mask, cv2.COLOR_BGR2GRAY)
mask = alpha.astype(np.uint8)
# 将原始图像和透明图像合并
result = np.concatenate((frame, mask), axis=1)
# 显示结果
cv2.imshow("Result", result)
# 按下q键退出循环
if cv2.waitKey(1) == ord("q"):
break
# 释放摄像头并关闭窗口
cap.release()
cv2.destroyAllWindows()
```
代码中使用了dlib的人脸检测器和关键点检测器来检测人脸并定位嘴巴内部的关键点,然后使用OpenCV创建一个透明图像,将嘴巴内部的轮廓填充为白色并绘制在透明图像上,最后将原始图像和透明图像合并。运行代码后,摄像头会实时显示出嘴巴内部轮廓彩色图片,轮廓外部为透明。
阅读全文
相关推荐
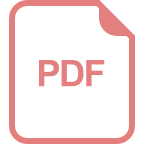






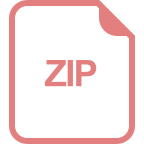
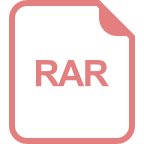
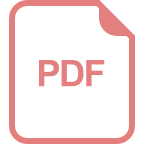
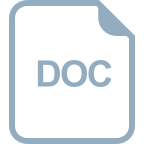
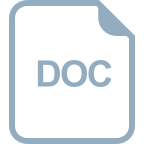


