for in/for of
时间: 2023-10-20 18:08:05 浏览: 68
`for in` and `for of` are both loops used in JavaScript for iteration, but they have different use cases and syntax.
`for in`:
The `for in` loop is used for iterating over the properties of an object. It is generally used with objects, and not with arrays or strings. The syntax of `for in` is:
```
for (variable in object) {
// code to be executed
}
```
Here, `variable` represents a property name of the object, and `object` is the object being iterated. The loop will iterate over all the enumerable properties of the object, including those inherited from its prototype chain.
Example:
```
const person = {
name: 'John',
age: 30,
gender: 'male'
};
for (let property in person) {
console.log(`${property}: ${person[property]}`);
}
// Output:
// name: John
// age: 30
// gender: male
```
`for of`:
The `for of` loop is used for iterating over the values of an iterable object, such as an array or a string. The syntax of `for of` is:
```
for (variable of iterable) {
// code to be executed
}
```
Here, `variable` represents the value of each element in the iterable, and `iterable` is the iterable object being iterated. The loop will iterate over all the elements of the iterable, in the order they appear.
Example:
```
const colors = ['red', 'green', 'blue'];
for (let color of colors) {
console.log(color);
}
// Output:
// red
// green
// blue
```
In summary, `for in` is used for iterating over properties of an object, while `for of` is used for iterating over values of an iterable object.
阅读全文
相关推荐
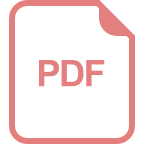
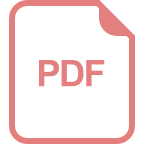
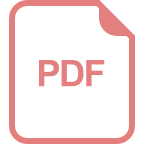
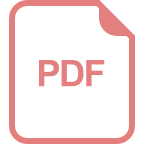
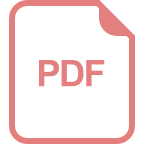
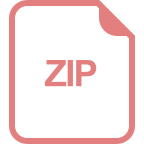
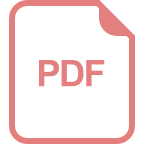
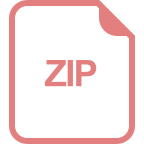
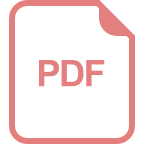
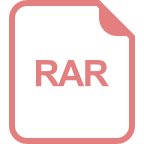
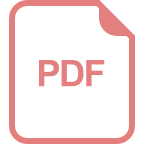
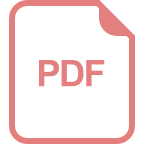
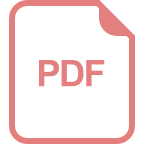
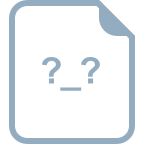
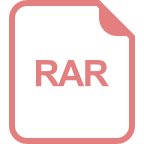
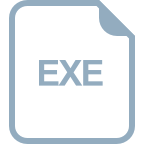
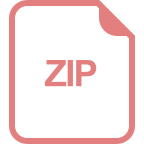