C# int 类型整数200转字节数组
时间: 2024-09-29 12:01:33 浏览: 11
在C#中,你可以使用BitConverter类将int类型的整数转换为字节数组。以下是一个示例:
```csharp
using System;
using System.Runtime.InteropServices;
public class Program
{
public static void Main()
{
int value = 200; // 要转换的整数值
// 创建一个byte数组来存放int值的四个字节
byte[] bytes = BitConverter.GetBytes(value);
Console.WriteLine("Original Value: " + value);
Console.WriteLine("Byte Array: [" + string.Join(", ", bytes) + "]");
}
}
```
运行这段代码,你会看到输出类似于这样的结果:
```
Original Value: 200
Byte Array: [0x00, 0x00, 0x00, 0xC8]
```
这里,`BitConverter.GetBytes(int)`函数返回一个包含4个字节(因为int占32位)的数组,高位字节放在数组的第一个元素。
相关问题
C#整数数组转换成字节数组
可以使用BitConverter类将整数数组转换为字节数组。以下是示例代码:
```csharp
int[] intArray = { 1, 2, 3, 4, 5 };
byte[] byteArray = new byte[intArray.Length * sizeof(int)];
Buffer.BlockCopy(intArray, 0, byteArray, 0, byteArray.Length);
```
这里使用了`Buffer.BlockCopy`方法将整数数组中的数据复制到字节数组中。`sizeof(int)`返回一个整数类型的字节大小,因此可以使用`intArray.Length * sizeof(int)`来计算字节数组的长度。注意,这种方法只适用于使用相同的平台和编译器的程序之间的数据传输。如果需要跨平台传输数据,则需要考虑字节序等因素。
C#整数数组转换成字节数组有哪些方法
在C#中,将整数数组转换为字节数组有以下几种方法:
1. 使用BitConverter类的GetBytes方法:
```csharp
int[] intArray = { 1, 2, 3 };
byte[] byteArray = new byte[intArray.Length * sizeof(int)];
Buffer.BlockCopy(intArray, 0, byteArray, 0, byteArray.Length);
```
2. 使用BitConvert.GetBytes方法:
```csharp
int[] intArray = { 1, 2, 3 };
byte[] byteArray = new byte[intArray.Length * sizeof(int)];
for (int i = 0; i < intArray.Length; i++)
{
byte[] tempArray = BitConverter.GetBytes(intArray[i]);
Array.Copy(tempArray, 0, byteArray, i * sizeof(int), sizeof(int));
}
```
3. 使用MemoryMarshal.TryWrite方法:
```csharp
int[] intArray = { 1, 2, 3 };
byte[] byteArray = new byte[intArray.Length * sizeof(int)];
for (int i = 0; i < intArray.Length; i++)
{
MemoryMarshal.TryWrite(byteArray.AsSpan(i * sizeof(int)), ref intArray[i]);
}
```
其中,第一种方法使用了Buffer.BlockCopy方法,可以将整个int数组转换为字节数组,而后两种方法需要遍历整个数组并将每个int值转换为字节数组。第三种方法使用了MemoryMarshal.TryWrite方法,这是C# 7.2中新增的方法,用于将值类型写入内存中。
相关推荐
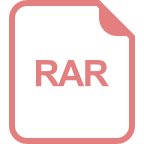
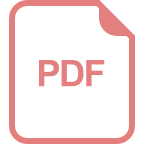
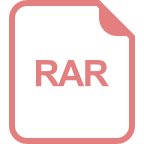












