#include<stdio.h> #include<stdlib.h> #include<time.h> #define MAX_SIZE 1000 typedef struct{ int key; }element; element list [MAX_SIZE]; void insertionSort(int r[], int n) { int p,j; for(p = 1; p < n; p++) { int tmp = r[p]; for(j = p; j > 0 && tmp < r[j-1]; j--) r[j + 1] = r [j]; r[j + 1] = tmp; } } void shellSort(int r[], int n) { int i, j, gap; int tmp; for(int gap = n / 2; gap > 0; gap /= 2) for(i = gap; i< n; i++){ tmp = r[i]; for(j = i; j >= gap && r[j-gap]>tmp; j-=gap) r[j] = r[j-gap]; r[j] = tmp; } } int main() { int r[50],i; { srand(time(NULL)); for(i = 0; i < 50; i++) r[i] = rand(); } { shellSort(r,50); } }
时间: 2024-04-27 12:22:01 浏览: 92
这段代码是一个C语言程序,实现了希尔排序(shellSort)和插入排序(insertionSort)两种排序算法。程序中使用了结构体(element)和数组(list)来存储数据。在主函数(main)中,首先生成了50个随机数,然后使用希尔排序对这些随机数进行排序。希尔排序的原理是先将数据按照一定间隔(gap)分组,对每组进行插入排序,然后逐渐缩小间隔,重复进行分组和排序操作,直到最后间隔为1时进行插入排序完成整个排序过程。插入排序的原理是将数据分为已排序区和未排序区,每次从未排序区选取一个元素插入到已排序区的合适位置,直到未排序区为空为止。
相关问题
#include <stdio.h> #include <stdlib.h> #define MAXSIZE 100 typedef int ElemType; typedef struct
这段代码是C语言中的头文件引用和一些定义的示例。其中,`#include <stdio.h>`和`#include <stdlib.h>`是用来引入标准库函数的头文件。`stdio.h`包含了输入输出相关的函数,如`printf`和`scanf`;`stdlib.h`包含了一些常用的函数,如内存分配函数`malloc`和随机数生成函数`rand`。
`#define MAXSIZE 100`是一个宏定义,用来定义一个常量`MAXSIZE`,它的值为100。
`typedef int ElemType;`是用来给`int`类型起一个别名`ElemType`,方便在代码中使用。
至于代码中的结构体部分,由于你没有提供完整的代码,所以无法给出具体的解释。但一般来说,结构体用于定义一种自定义的数据类型,可以包含多个不同类型的成员变量。
#include <stdio.h> #include <stdlib.h> #include <stdbool.h> #define MaxSize 100 //顺序栈
`#include <stdio.h>`、`#include <stdlib.h>` 和 `#include <stdbool.h>` 这些头文件在C语言程序中分别引入了标准输入输出库(<stdio.h>)、内存管理库(<stdlib.h>)以及布尔类型支持(<stdbool.h>)。`MaxSize 100` 定义了一个常量,表示顺序栈的最大容量为100,通常用于限制栈元素的数量。
顺序栈是一种基于数组实现的数据结构,它按照先进后出(LIFO,Last In First Out)的原则存储和访问数据。在C中,你可以创建一个数组来模拟栈的行为,例如用数组下标作为栈顶指针,当栈满时插入操作会溢出,当栈空时弹出操作会访问到无效位置。
下面是一个简单的顺序栈实现示例:
```c
typedef struct Stack {
int* data; // 存储栈元素的数组
int top; // 栈顶指针
int size; // 栈的实际大小
} Stack;
Stack* createStack() { // 创建栈函数
Stack* stack = (Stack*) malloc(sizeof(Stack));
if (!stack) {
perror("Memory allocation failed");
exit(EXIT_FAILURE);
}
stack->data = (int*) malloc(MaxSize * sizeof(int));
if (!stack->data) {
free(stack); // 如果内存分配失败释放已经分配的部分
perror("Memory allocation failed");
exit(EXIT_FAILURE);
}
stack->top = -1;
stack->size = MaxSize;
return stack;
}
bool push(Stack* stack, int value) { // 入栈操作
if (stack->top == stack->size - 1) {
printf("Stack overflow.\n");
return false;
}
stack->data[++stack->top] = value;
return true;
}
int pop(Stack* stack) { // 出栈操作
if (stack->top == -1) {
printf("Stack underflow.\n");
return -1; // 返回特殊值表示栈空
}
return stack->data[stack->top--];
}
void destroyStack(Stack* stack) { // 销毁栈并释放内存
free(stack->data);
free(stack);
}
```
阅读全文
相关推荐
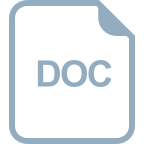
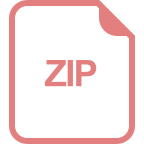













