stm32温度采集AHT10
时间: 2023-09-10 12:11:04 浏览: 246
AHT10是一款数字温湿度传感器,可以通过I2C接口与STM32进行通信。以下是基本的步骤:
1. 配置I2C接口:选择正确的引脚并使能I2C时钟。
2. 初始化AHT10传感器:发送启动命令,等待传感器响应,并设置传感器的采样模式和测量范围。
3. 读取温度和湿度数据:发送读取命令并等待传感器响应,然后读取传感器返回的数据并将其转换为温度和湿度值。
具体的代码实现可以参考以下示例:
```c
#include "stm32f4xx.h"
#include "stdlib.h"
#include "stdio.h"
#include "string.h"
#include "math.h"
#define AHT10_ADDR 0x38
I2C_HandleTypeDef hi2c1;
void SystemClock_Config(void);
static void MX_GPIO_Init(void);
static void MX_I2C1_Init(void);
void AHT10_Init(void)
{
uint8_t buf[3] = {0x08, 0x00, 0x00};
HAL_I2C_Master_Transmit(&hi2c1, AHT10_ADDR<<1, buf, 3, 100);
}
void AHT10_Start(void)
{
uint8_t buf[1] = {0xAC};
HAL_I2C_Master_Transmit(&hi2c1, AHT10_ADDR<<1, buf, 1, 100);
}
void AHT10_Read(float *temp, float *humid)
{
uint8_t buf[6];
HAL_I2C_Master_Receive(&hi2c1, AHT10_ADDR<<1, buf, 6, 100);
uint32_t raw_humid = ((uint32_t)buf[1] << 12) | ((uint32_t)buf[2] << 4) | ((uint32_t)buf[3] >> 4);
uint32_t raw_temp = (((uint32_t)buf[3] & 0x0F) << 16) | ((uint32_t)buf[4] << 8) | buf[5];
*temp = (float)raw_temp * 200.0 / 1048576.0 - 50.0;
*humid = (float)raw_humid * 100.0 / 1048576.0;
}
int main(void)
{
float temp, humid;
HAL_Init();
SystemClock_Config();
MX_GPIO_Init();
MX_I2C1_Init();
AHT10_Init();
while (1)
{
AHT10_Start();
HAL_Delay(100);
AHT10_Read(&temp, &humid);
printf("Temperature: %.2f C, Humidity: %.2f %%\r\n", temp, humid);
HAL_Delay(1000);
}
}
void SystemClock_Config(void)
{
RCC_OscInitTypeDef RCC_OscInitStruct = {0};
RCC_ClkInitTypeDef RCC_ClkInitStruct = {0};
__HAL_RCC_PWR_CLK_ENABLE();
__HAL_PWR_VOLTAGESCALING_CONFIG(PWR_REGULATOR_VOLTAGE_SCALE1);
RCC_OscInitStruct.OscillatorType = RCC_OSCILLATORTYPE_HSI;
RCC_OscInitStruct.HSIState = RCC_HSI_ON;
RCC_OscInitStruct.HSICalibrationValue = RCC_HSICALIBRATION_DEFAULT;
RCC_OscInitStruct.PLL.PLLState = RCC_PLL_ON;
RCC_OscInitStruct.PLL.PLLSource = RCC_PLLSOURCE_HSI;
RCC_OscInitStruct.PLL.PLLM = 8;
RCC_OscInitStruct.PLL.PLLN = 180;
RCC_OscInitStruct.PLL.PLLP = RCC_PLLP_DIV2;
RCC_OscInitStruct.PLL.PLLQ = 4;
if (HAL_RCC_OscConfig(&RCC_OscInitStruct) != HAL_OK)
{
Error_Handler();
}
RCC_ClkInitStruct.ClockType = RCC_CLOCKTYPE_HCLK|RCC_CLOCKTYPE_SYSCLK
|RCC_CLOCKTYPE_PCLK1|RCC_CLOCKTYPE_PCLK2;
RCC_ClkInitStruct.SYSCLKSource = RCC_SYSCLKSOURCE_PLLCLK;
RCC_ClkInitStruct.AHBCLKDivider = RCC_SYSCLK_DIV1;
RCC_ClkInitStruct.APB1CLKDivider = RCC_HCLK_DIV4;
RCC_ClkInitStruct.APB2CLKDivider = RCC_HCLK_DIV2;
if (HAL_RCC_ClockConfig(&RCC_ClkInitStruct, FLASH_LATENCY_5) != HAL_OK)
{
Error_Handler();
}
}
static void MX_I2C1_Init(void)
{
hi2c1.Instance = I2C1;
hi2c1.Init.ClockSpeed = 100000;
hi2c1.Init.DutyCycle = I2C_DUTYCYCLE_2;
hi2c1.Init.OwnAddress1 = 0;
hi2c1.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT;
hi2c1.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE;
hi2c1.Init.OwnAddress2 = 0;
hi2c1.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE;
hi2c1.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE;
if (HAL_I2C_Init(&hi2c1) != HAL_OK)
{
Error_Handler();
}
}
static void MX_GPIO_Init(void)
{
GPIO_InitTypeDef GPIO_InitStruct = {0};
__HAL_RCC_GPIOB_CLK_ENABLE();
HAL_GPIO_WritePin(GPIOB, GPIO_PIN_6|GPIO_PIN_7, GPIO_PIN_SET);
GPIO_InitStruct.Pin = GPIO_PIN_6|GPIO_PIN_7;
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP;
GPIO_InitStruct.Pull = GPIO_NOPULL;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW;
HAL_GPIO_Init(GPIOB, &GPIO_InitStruct);
}
```
在这个示例代码中,我们使用了STM32的I2C1接口,将AHT10传感器连接到了PB6和PB7引脚上。在主循环中,我们不断地读取温度和湿度数据,并通过串口打印出来。需要注意的是,为了正确地读取AHT10传感器的数据,我们需要在每次读取数据之前发送一个启动命令。此外,AHT10传感器的温度和湿度数据都是原始数据,需要进行一定的计算才能得到实际的温度和湿度值。
阅读全文
相关推荐
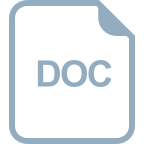
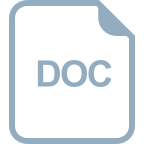
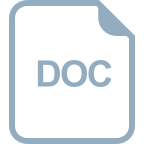

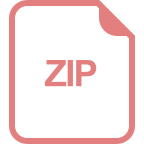

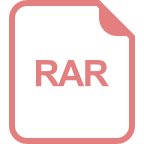
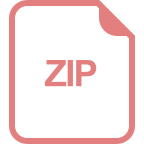
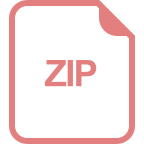
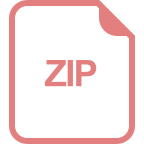
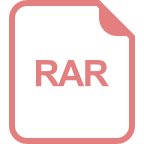
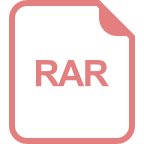
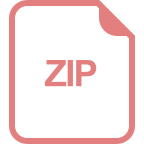
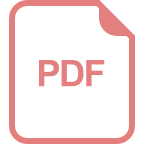
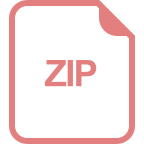
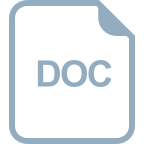
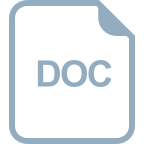
